The Ultimate Guide to React Native Video Recording
Looking to add video recording capabilities to your React Native app? Look no further! This guide will show you everything you need to know about React Native video recording, including how to set up your environment, capture video from the camera, and render it to the user.
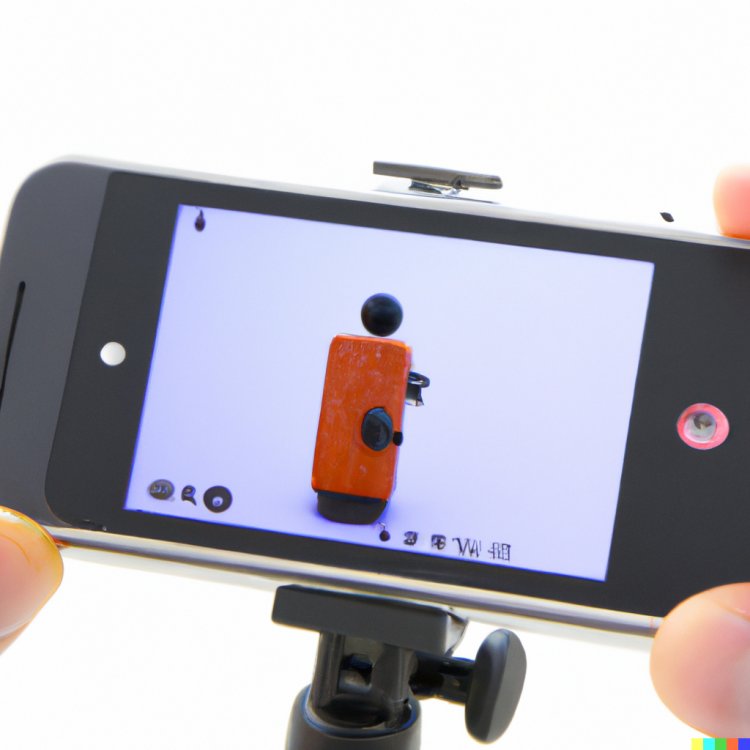
Introduction to React Native Video Recording
React Native is a JavaScript framework for building native mobile apps. It’s based on React, a JavaScript library for building user interfaces, but instead of using web components, it uses native components.
This means that you can use all the React Native components, but you also have access to the native components of the platform you’re running on.
React Native also allows you to write code that’s specific to the platform you’re targeting. So, if you want to write a React Native app for iOS, you can use the same code on an Android device.
React Native provides a number of built-in components, such as View, Text, and Image. You can also create your own custom components.
React Native also has a number of third-party components that you can use.
Why You Might Want to Record Video in React Native
There are a few reasons you might want to record video in React Native. Maybe you're building a video app and you want to offer your users the ability to record and share videos. Or, maybe you're building a marketing or training app and you want to include video content. Whatever the reason, recording video in React Native is a great way to add engaging content to your app.
There are a few different ways to record video in React Native. You can use the built-in camera component, or you can use a third-party library like react-native-camera. Whichever method you choose, recording video in React Native is relatively straightforward.
If you're building a video app, you'll probably want to offer your users the ability to record and share videos. The built-in camera component makes this easy to do. Simply add the component to your app and allow your users to record videos. Once they're done recording, they can share the video with their friends or post it to social media.
If you're looking to add video content to your marketing or training app, you'll want to use a third-party library like react-native-camera. This library provides a more robust video recording experience, complete with the ability to record in high definition. Plus, with the react-native-camera library, you can also add video filters and effects, giving your videos a professional look and feel.
Recording video in React Native is a great way to add engaging content to your app. Whether you're looking to build a video app or add video content to your marketing or training app, there's a solution that's right for you. So, what are you waiting for? Start recording today!
Setting Up Your React Native Environment for Video Recording
If you're looking to get started with video recording in React Native, there are a few things you'll need to set up first. In this blog post, we'll walk through setting up your environment for recording video in React Native.
Before we get started, you'll need to make sure you have the following installed and set up:
- React Native
- Expo
- An iOS or Android device
Once you have all of that set up, you're ready to start recording video in React Native!
There are two ways to record video in React Native: using the Expo Camera API or the React Native CameraRoll API. We'll cover both methods in this blog post.
Using the Expo Camera API
To use the Expo Camera API, you'll first need to import the Camera component from the expo package.
import { Camera } from 'expo';
Once you have the Camera component imported, you can use it in your component's render() method like so:
render() {
return ();
}
This will render a basic camera view in your app. You can then add the following props to the Camera component to start recording video:
- type: 'video' (this will tell the camera to start recording video)
- onVideoRecorded: this is a function that will be called when the video has finished recording
Here's an example of how you would use the type and onVideoRecorded props:
render() {
return ();
}
The handleVideoRecorded function will be where you put your logic for what happens when the video has finished recording. In this function, you can do things like save the video to your device's storage, upload it to a server, etc.
Using the React Native CameraRoll API
The other way to record video in React Native is by using the CameraRoll API. This API allows you to access the videos stored on your device's camera roll.
To use the CameraRoll API, you'll first need to import the CameraRoll package from the expo package.
import { CameraRoll } from 'expo';
Once you have the CameraRoll package imported, you can use it in your component's render() method like so:
render() {
return ();
}
This will render a basic camera roll view in your app. You can then add the following props to the CameraRoll component to start recording video:
- mediaType: 'video' (this will tell the camera roll to only display videos)
- onSelect: this is a function that will be called when you select a video from the camera roll
Here's an example of how you would use the mediaType and onSelect props:
render() {
return ();
}
The handleVideoSelected function will be where you put your logic for what happens when a video is selected from the camera roll. In this function, you can do things like save the video to your device's storage, upload it to a server, etc.
And that's it! You now know how to set up your environment for recording video in React Native.
The React Native Camera Package
If you're looking to add a camera feature to your React Native app, you might be wondering what package to use. There are a few different options out there, but the most popular one is probably the React Native Camera package.
This package makes it easy to add a camera view to your React Native app, and it also provides a number of features like face detection and barcode scanning. In this blog post, we'll take a look at how to use the React Native Camera package to add a camera view to your app. We'll also learn about some of the other features that the package offers.
So let's get started!
Basic Video Recording in React Native
React Native is a JavaScript framework for building mobile applications using React. To record a video in a React Native app, you can use the react-native-camera module, which provides a camera component that can be used to record video. This can be installed using the following command:
npm install react-native-camera
After installation, you can import the Camera
component and use it to render a camera view in your app. You can then use the recordAsync
method to start and stop video recording. Here is an example of how you might use the Camera
component to record a video:
import React, { useState } from 'react';
import { TouchableOpacity, View } from 'react-native';
import { Camera } from 'react-native-camera';export default function App() {
const [isRecording, setIsRecording] = useState(false);
let cameraRef = useRef(null);const startRecording = async () => {
setIsRecording(true);
const video = await cameraRef.recordAsync();
console.log(video);
};const stopRecording = () => {
setIsRecording(false);
cameraRef.stopRecording();
};return (
<View style={{ flex: 1 }}>
<Camera style={{ flex: 1 }} ref={ref => { cameraRef = ref; }} />
<View style={{ flex: 0, flexDirection: 'row', justifyContent: 'center' }}>
<TouchableOpacity onPress={isRecording ? stopRecording : startRecording}>
<Text style={{ fontSize: 14, marginBottom: 10, color: 'white' }}>
{isRecording ? 'Stop Recording' : 'Start Recording'}
</Text>
</TouchableOpacity>
</View>
</View>
);
}
Conclusion
React Native is a great tool for creating mobile apps. It has many features that make it a great choice for developers, including the ability to record video.
The video recording feature in React Native is a great way to capture memories or Create a video tutorial. It is easy to use and provides high-quality video recordings.