Use React Hooks with the State Hook for Easier State Management | React hooks
React state management can be tricky, but the React hooks API makes it easier. The useState hook allows you to quickly and easily access and manipulate your application's state. Learn how to use it for better state management in your React applications.
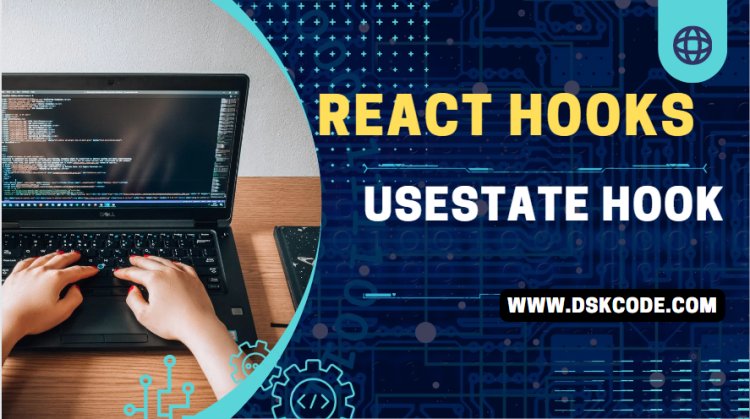
Hooks are a new feature introduced in React 16.8 that allow you to use state and other React features without writing a class.
The useState hook is a way to add state to a functional component. To use the useState hook, you first need to import it into your file. Then you can declare a state variable and a setter function with the useState() method.
The useState() method takes in an initial state as an argument. You can then use the state variable in your component. To update the state, you can use the setter function that is returned by the useState() method.
Hooks are a new feature in React 16.8 that lets you use state and other React features without writing a class.
The useState hook allows you to add state to functional components.
Table of Content
1. Import useState from React
2. Declare a state variable and a setter function with useState
3. Use the state variable in your component
4. Update the state with the setter function
Import useState from React
import React, { useState } from 'react';
const Example = () => {
// Declare a new state variable, which we'll call "count"
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Example;
The useState() hook is a function that takes a single argument, which is the initial state. It returns an array with two items: the current state (which is equivalent to this.state in a class) and a function that updates it (which is equivalent to this.setState in a class).
In the example above, we declared a state variable called count and set its initial value to 0. We then returned a element that displays the current count. Finally, we added a button that calls the setCount() function when clicked. Every time the button is clicked, the setCount() function is called with the current count + 1.
Declare a state variable and a setter function with useState
const [stateVariable, setStateVariable] = useState();
// Example:
const [name, setName] = useState('John Doe');// Setting a new state
setName('Jane Doe');
Use the state variable in your component
React Hooks useState is a built-in hook that allows components to have local state. It is a function that takes in an initial state and returns an array of two values, the current state and a function to update it.
Example:
const Example = () => {
const [count, setCount] = useState(0);return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}export default Example;
export default Example;
Update the state with the setter function
React Hooks useState is a built-in hook that allows you to manage state in your React components. To use it, you pass in a single argument, which is the initial value of the state.
For example, if you wanted to store a boolean value of whether a user is logged in or not, you could use the useState hook like this:
const [isLoggedIn, setIsLoggedIn] = useState(false);
This declares a new state variable called "isLoggedIn" with the initial value of false. The setIsLoggedIn function is used to update the state variable. So, if you wanted to update the state variable to true, you would call the setIsLoggedIn function like so:
setIsLoggedIn(true);
Powerful Calculator App for All Your Math Needs
This powerful calculator app makes solving math problems easier than ever before. With an easy-to-use interface and intuitive design, you can quickly and accurately compute complex equations. Plus, the app includes a range of features like a graphing calculator, memory functions, and an equation solver, so you can easily complete any task. Whether you're a student, a professional, or just a casual user, this app has everything you need to solve any math problem. Get the most out of your math experience today with this powerful calculator app!
import React, { useState } from "react";
const Calculator = () => {
// Declare a new state variable, which we'll call "count"
const [count, setCount] = useState(0);
const [num1, setNum1] = useState(0);
const [num2, setNum2] = useState(0);
const [result, setResult] = useState(0);const addNumbers = () => {
setResult(parseInt(num1) + parseInt(num2));
}const subtractNumbers = () => {
setResult(parseInt(num1) - parseInt(num2));
}const multiplyNumbers = () => {
setResult(parseInt(num1) * parseInt(num2));
}const divideNumbers = () => {
setResult(parseInt(num1) / parseInt(num2));
}return (
<div>
<input type="text" value={num1} onChange={(e) => setNum1(e.target.value)} />
<input type="text" value={num2} onChange={(e) => setNum2(e.target.value)} />
<button onClick={addNumbers}>+</button>
<button onClick={subtractNumbers}>-</button>
<button onClick={multiplyNumbers}>*</button>
<button onClick={divideNumbers}>/</button>
<h3>{result}</h3>
</div>
);
};
Example use of the useState Hook
The useState Hook allows us to store a piece of state in our component. In this example, we are using the Hook to create a simple calculator. We declare four state variables to store the numbers we want to calculate, the result of the calculation, and the count of how many times the calculation has been performed. We then create four functions to perform the operations of addition, subtraction, multiplication, and division, and use the setResult function to update the result state variable. Finally, we render the input fields, the buttons to perform the calculations, and the result.