Leveraging Passport JS for Authentication in Node JS
Passport.js is an authentication middleware for Node.js that can be used to authenticate users with a wide variety of authentication strategies. It provides an easy-to-use framework for integrating authentication into any application, allowing you to quickly and securely authenticate users with a variety of credentials. Leverage Passport.js for authentication in Node.js and secure your application!
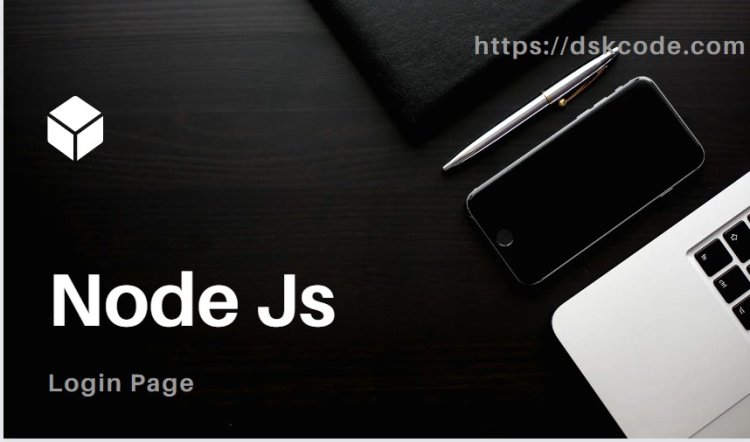
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine. It is used to develop server-side applications, create network applications and build web applications. Node.js enables developers to create scalable, data-intensive and real-time applications with ease.
A Node.js login page is an essential part of any web application, allowing users to create accounts and log in securely. It usually consists of a username and password field, along with a Submit button. It may also include other features such as a captcha to prevent automated bots from accessing the system. The login page should be designed in a way that is both secure and user-friendly. A properly designed login page can also be used to prompt users to make updates to their profiles or to provide other useful information.
Here's a basic example of a login page using Node.js, Express, and Passport for authentication. This example uses local strategy for username and password authentication, and stores user data in an array for simplicity.
Step 1: Set up a new Node.js project and install necessary dependencies. First, create a new directory for your project and navigate into it using the command line. Then, run the following commands:
npm install express passport passport-local express-session
This will create a new Node.js project and install Express, Passport, Passport-local, and Express-session dependencies.
Step 2: Create an Express application and configure it. Create a new file called app.js in your project directory and add the following code:
// Import dependencies
const express = require('express');
const passport = require('passport');
const LocalStrategy = require('passport-local').Strategy;
const session = require('express-session');
// Create an Express app
const app = express();
// Configure Passport
passport.use(new LocalStrategy((username, password, done) => {
// Here, you can add your own logic for checking if the username and password are valid
// For simplicity, we'll use a hardcoded user array
const users = [
{ id: 1, username: 'user1', password: 'password1' },
{ id: 2, username: 'user2', password: 'password2' },
];
const user = users.find(u => u.username === username && u.password === password);
if (!user) {
return done(null, false, { message: 'Invalid username or password' });
}
return done(null, user);
}));
passport.serializeUser((user, done) => {
done(null, user.id);
});
passport.deserializeUser((id, done) => {
// Here, you can fetch user data from your database based on the serialized user id
// For simplicity, we'll use the hardcoded user array
const users = [
{ id: 1, username: 'user1', password: 'password1' },
{ id: 2, username: 'user2', password: 'password2' },
];
const user = users.find(u => u.id === id);
done(null, user);
});
// Configure Express middleware
app.use(express.urlencoded({ extended: true }));
app.use(express.json());
app.use(session({
secret: 'your-secret-key', // Replace with your own secret key
resave: false,
saveUninitialized: false,
}));
app.use(passport.initialize());
app.use(passport.session());
// Define routes
app.get('/', (req, res) => {
res.send('Welcome to the login page!');
});
app.post('/login', passport.authenticate('local', {
successRedirect: '/dashboard', // Redirect to dashboard on successful login
failureRedirect: '/login', // Redirect to login page on failed login
}));
app.get('/dashboard', (req, res) => {
// Access user data using req.user
res.send(`Welcome to the dashboard, ${req.user.username}!`);
});
app.get('/login', (req, res) => {
res.send('Please login');
});
// Start the server
app.listen(3000, () => {
console.log('Server started on http://localhost:3000');
});
Step 3: Run the server and test the login functionality Run the following command in your project directory to start the server:
Tips & Tricks Login
Node.js is an open source and cross-platform runtime environment for executing JavaScript code outside of a web browser. It provides an easy way to create web applications and can be used to build complex server-side applications. Having a secure login page is essential for any web application, and here are some tips and tricks to help you get the most out of your Node.js login page:
1. Use an authentication library: It is recommended to use a third-party authentication library such as Passport.js to handle user authentication in Node.js. This will help to ensure that all authentication is secure and the credentials are stored properly.
2. Use HTTPS: HTTPS should be used for all login pages. This is to ensure that the data submitted is encrypted and secure.
3. Use strong passwords: Passwords should be strong and hard to guess. Make sure to use a combination of numbers, letters, and special characters.
4. Use two-factor authentication: Two-factor authentication adds an extra layer of security by requiring two pieces of information from the user in order to log in.
5. Use a captcha: Using a captcha on the login page can help to prevent automated attempts to guess passwords.
6. Log failed attempts: It is important to log failed login attempts and alert the user if there are too many failed attempts.
7. Limit login attempts: Limit the number of login attempts per user to prevent brute-force attacks.
8. Use an access token: An access token is used to grant access to a resource. It should be stored securely and not passed in a query string.
9. Log out inactive users: Log out users who have been inactive for a certain period of time. This is to prevent users from leaving their accounts open and vulnerable to attack.
10. Use SSL: SSL should be used to encrypt communication between the client and server. This will help ensure that sensitive data is not exposed.