Optimize Image Loading in React Native Fast Image
Improve your React Native app's performance with React Native Fast Image. Customizable and efficient image loading for a seamless experience.
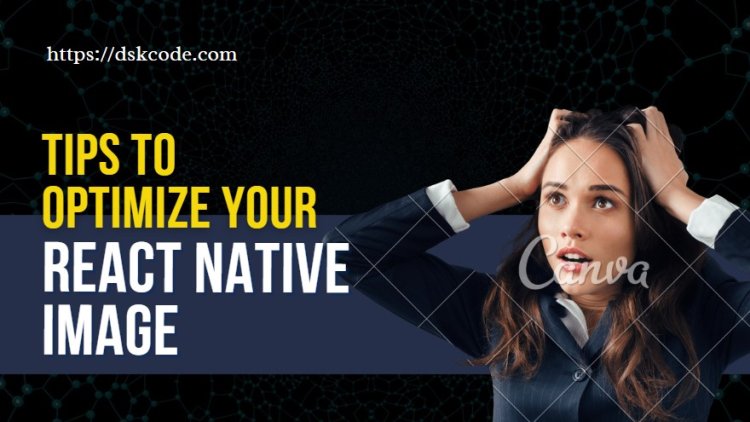
Table Of Content
I. Introduction
II. Step by Step Guide with Explanations
III. Features of React Native Fast Image
IV. Benefits of React Native Fast Image
V. Limitations of React Native Fast Image
VI. Conclusion
Introduction
When it comes to image caching in React Native, the React Native Fast Image library is the go-to solution. With its customizable image loading capabilities, React Native developers can easily optimize image loading in their applications.
The Fast Image component is specifically designed to provide efficient image loading in React Native. It uses image caching techniques to minimize the need for repeated image downloads, resulting in faster loading times and improved performance. Developers can customize various aspects of image loading, such as image quality, priority, and placeholders, to suit their specific requirements.
By incorporating the Fast Image component into their React Native projects, developers can significantly enhance the user experience by delivering high-quality images quickly and seamlessly. With its optimized image loading capabilities, the Fast Image library is a valuable tool for any React Native developer looking to boost their app's performance and provide a smooth and efficient image loading experience for their users.
Step by Step Guide with Explanations
Step 1: Install React Native Fast Image
You can install React Native Fast Image using npm or yarn. Open your terminal and run the following command:
or
Step 2: Link Native Dependencies
React Native Fast Image requires linking native dependencies to the underlying platform (iOS and Android). You can use the following commands to link the dependencies:
For iOS:
For Android
Step 3: Import React Native Fast Image You need to import React Native Fast Image in your React Native component where you want to use it. You can do this by adding the following import statement:
Step 4: Use React Native Fast Image in Your Component
You can now use the FastImage component in your React Native component. Here's an example of how you can use it:
import { View, Image, StyleSheet } from 'react-native';
import FastImage from 'react-native-fast-image';
const MyImageComponent = () => {
return (
<View style={styles.container}>
<FastImage
style={styles.image}
source={{
uri: 'https://example.com/my-image.jpg',
priority: FastImage.priority.normal,
}}
resizeMode={FastImage.resizeMode.contain}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
image: {
width: 200,
height: 200,
},
});
export default MyImageComponent;
In this example, we're using the FastImage component to display an image from a remote URL. We've provided the image URL as the uri prop in the source prop. We've also set the resizeMode prop to 'contain' to ensure that the image maintains its aspect ratio and fits within the specified dimensions.
Step 5: Handle Error Cases
React Native Fast Image also provides error handling capabilities. You can handle different error scenarios, such as image load failure, by using the onError prop.
style={styles.image}
source={{
uri: 'https://example.com/my-image.jpg',
priority: FastImage.priority.normal,
}}
resizeMode={FastImage.resizeMode.contain}
onError={() => console.log('Image load failed')}
/>
if the image fails to load, the onError callback will be triggered, and you can handle the error as needed, such as showing a placeholder image or displaying an error message.
Features of React Native Fast Image
React Native Fast Image offers several powerful features that make it a popular choice for handling images in React Native applications:
- Image Caching: React Native Fast Image automatically caches images, allowing for efficient loading and rendering of images even when offline or in low network conditions. This helps improve app performance and reduce unnecessary network requests.
- Image Prefetching: The library provides an easy way to prefetch images in the background, allowing developers to load images ahead of time and provide a faster and smoother image loading experience for users.
- Progressive Image Loading: React Native Fast Image supports progressive image loading, which allows images to be loaded in multiple passes, starting with a lower-quality placeholder image and gradually transitioning to higher-quality versions. This helps improve perceived loading speed and user experience.
- Advanced Image Resizing and Transformation: The library offers various image resizing and transformation options, such as resizing, cropping, rotating, and applying filters, allowing developers to easily manipulate and display images in different sizes, formats, and styles.
- Image Source Flexibility: React Native Fast Image supports a wide range of image sources, including local files, remote URLs, and assets bundled with the app. This gives developers flexibility in choosing image sources that best fit their application's needs.
- Customization: The library is highly customizable, allowing developers to configure various settings such as caching strategy, loading indicators, error handling, and more, to suit their specific requirements and design preferences.
- Performance Optimization: React Native Fast Image is designed to be performant, with features such as automatic image decoding and memory management, optimized image loading algorithms, and efficient handling of large images, all contributing to improved performance and smoother image loading experiences.
- Error Handling: The library provides comprehensive error handling, including customizable error states and retry mechanisms, making it easy to handle cases where images fail to load due to network issues or other errors.
- Seamless Integration: React Native Fast Image seamlessly integrates with the React Native ecosystem, making it easy to use in conjunction with other popular libraries and tools for building React Native applications.
Overall, React Native Fast Image offers a robust set of features that help developers optimize image loading performance, provide a smooth user experience, and easily handle various image-related tasks in React Native applications.
Benefits of React Native Fast Image
React Native Fast Image offers several benefits that make it a valuable tool for handling images in React Native applications:
- Improved Performance: React Native Fast Image is designed to optimize image loading and rendering performance, helping to deliver fast and smooth image loading experiences for users. Features such as image caching, prefetching, progressive loading, and advanced resizing help reduce image loading times, minimize network requests, and improve overall app performance.
- Seamless User Experience: Fast and efficient image loading is crucial for providing a seamless user experience in mobile apps. React Native Fast Image helps ensure that images load quickly and smoothly, minimizing the waiting time for users and enhancing the overall app usability.
- Customization Options: React Native Fast Image offers a high degree of customization, allowing developers to configure various settings such as caching strategy, image resizing, error handling, and more. This flexibility enables developers to tailor the library to their specific needs and design requirements.
- Image Source Flexibility: React Native Fast Image supports a wide range of image sources, including local files, remote URLs, and bundled app assets. This allows developers to easily handle different types of images, from local resources to images fetched from APIs, without needing to use multiple libraries or complex logic.
- Error Handling: React Native Fast Image provides comprehensive error handling features, including customizable error states and retry mechanisms, which helps handle cases where images fail to load due to network issues or other errors. This improves the user experience by gracefully handling error scenarios and providing meaningful feedback to users.
- Progressive Image Loading: Progressive image loading is a powerful feature of React Native Fast Image that allows images to be loaded in multiple passes, starting with a lower-quality placeholder image and gradually transitioning to higher-quality versions. This helps improve perceived loading speed and provides a smooth visual experience to users as images load.
- Easy Integration: React Native Fast Image integrates seamlessly with the React Native ecosystem, making it easy to use alongside other popular libraries and tools for building React Native applications. This simplifies the integration process and allows developers to leverage the benefits of the library without disrupting their existing development workflow.
- Active Community and Support: React Native Fast Image has a large and active community of developers who actively contribute to its development and provide support through documentation, forums, and GitHub issues. This ensures that developers have access to resources and assistance when using the library in their applications.
Overall, React Native Fast Image offers numerous benefits, including improved performance, seamless user experience, customization options, image source flexibility, error handling, progressive image loading, easy integration, and community support. These benefits make it a popular choice for handling images in React Native applications and can significantly enhance the image loading performance and user experience in mobile apps.
Limitations of React Native Fast Image
While React Native Fast Image provides many powerful features, it also has some limitations that developers should be aware of:
- Native Dependencies:
React Native Fast Image relies on native modules and requires linking native dependencies to the underlying platform (iOS and Android). This can introduce additional setup and configuration steps, and may require extra effort to maintain compatibility with different React Native versions or platforms.
- Platform-Specific Code:
React Native Fast Image may require platform-specific code to handle certain advanced features, such as prefetching and image transformation. This can increase the complexity of the codebase, and may require platform-specific knowledge and expertise.
- Limited Image Manipulation:
While React Native Fast Image provides basic image manipulation options such as resizing, cropping, and rotating, it may not have all the advanced image manipulation features that some other image processing libraries offer. For complex image manipulation tasks, additional third-party libraries or custom implementations may be required.
- Performance Considerations:
Although React Native Fast Image is designed to optimize image loading performance, the actual performance can still be affected by factors such as the size and resolution of images, network conditions, and device capabilities. Careful consideration and testing of image sizes, caching strategies, and other performance-related settings may be needed to ensure optimal performance.
- Image Format Support:
React Native Fast Image supports commonly used image formats such as JPEG, PNG, and GIF, but may not support less common or proprietary image formats. This may require additional image format conversion or handling in the app to ensure compatibility with React Native Fast Image.
- Development and Maintenance:
As with any open-source library, the development and maintenance of React Native Fast Image depend on the contributions and support of the community. While the library has a large and active community, updates and bug fixes may not be as frequent or timely as desired, and issues may take longer to be resolved.
- App Size:
React Native Fast Image adds to the app size as it includes native modules for iOS and Android. This may increase the overall size of the app, especially if the app uses other native modules as well. App size considerations should be taken into account, especially for apps with limited storage or slow network connections.
- Compatibility and Upgrades:
React Native Fast Image may have dependencies on specific versions of React Native or other libraries, and upgrades to newer versions of React Native or other dependencies may require additional effort to ensure compatibility. It's important to consider the potential impact on the app's stability and functionality when upgrading or making changes to dependencies.
Despite these limitations, React Native Fast Image remains a popular and widely used library for handling images in React Native applications, offering many benefits and features that can greatly enhance the image loading performance and user experience in mobile apps. It's important for developers to carefully evaluate their specific requirements and consider these limitations when choosing and using React Native Fast Image in their applications.
Conclusion
React Native Fast Image is a powerful and popular library for handling images in React Native applications. It offers numerous benefits, such as improved performance, seamless user experience, customization options, image source flexibility, error handling, progressive image loading, easy integration, and community support.
However, like any library, React Native Fast Image also has some limitations, including native dependencies, platform-specific code, limited image manipulation, performance considerations, image format support, development and maintenance, app size, and compatibility and upgrade considerations. Developers should carefully evaluate these limitations and consider their specific requirements before using React Native Fast Image in their applications.
Overall, React Native Fast Image can be a valuable tool for optimizing image loading performance and improving the user experience in React Native applications. By leveraging its features and benefits, developers can efficiently handle images in their apps and deliver fast and smooth image loading experiences to users.