Boost Your App's Performance with Image Compression
Improve the loading times of your React Native app by reducing image sizes. Enhance your React Native app's user experience with efficient image compression.
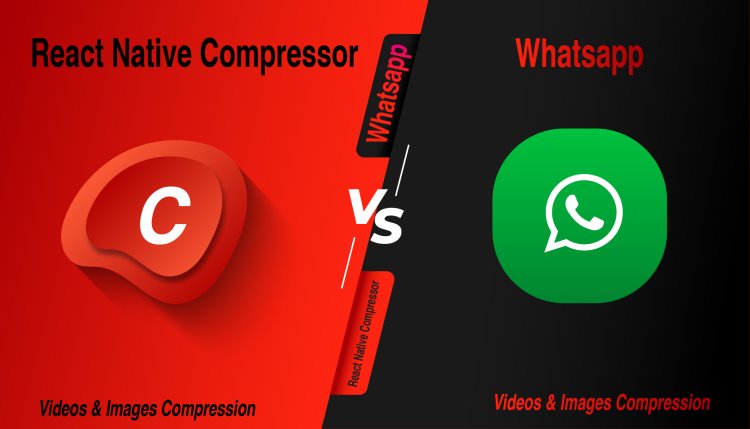
Table Of Content
I. Introduction
II. Choose an Image Compression Library
III. Compress the Image
IV. Save the Compressed Image
V. Display the Compressed Image
VI. Conclusion
I. Introduction
React Native image compress and react native compress image are techniques used in React Native to reduce the file size of images without compromising their quality or detail. The process involves reducing the number of colors and pixels used in an image, and optimizing it for faster loading times on mobile devices. By compressing images, developers can strike a balance between image quality and size, while also improving the user experience by reducing page loading times. Image compression is a crucial technique in React Native development, and by incorporating these techniques, developers can create faster, more efficient, and user-friendly applications.
React Native Image Compression is a powerful package designed to streamline the process of compressing images within React Native applications. This package enables developers to easily reduce the size of images without compromising their quality, while also minimizing memory usage within their applications. The package is compatible with both PNG and JPEG image formats and provides a range of customization options. With React Native Image Compression, developers can specify the desired level of compression and output size for their images, making it a versatile solution for various use cases. By leveraging the capabilities of this package, developers can optimize the performance of their React Native applications and provide users with a seamless and enjoyable experience.
II. Choose an Image Compression Library
The first step in compressing an image using React Native is to choose an image compression library. There are a number of popular libraries to choose from, such as React-Native-Compressor.
WINDOWS & MAC CMD
LINUX CMD
Developers to compress images on the fly within their React Native applications. Built on top of React Native Image Manipulator, this library provides a user-friendly API and an optional UI, making it easy to implement image compression functionality. It supports both iOS and Android platforms and is capable of compressing images of any size, including those larger than the device’s screen resolution. With support for multiple image formats, developers can easily control the size and quality of the compressed image using a few simple parameters. The library also includes several pre-defined compression presets, which can be used to quickly compress images to a specific size or quality, saving valuable time and effort. React Native Compressor is open source, and its developers actively maintain and update the library to ensure optimal performance and compatibility with the latest React Native releases.
Two Types of Image Compress
1. Automatic Image Compression Like Whatsapp
2. Manual Image Compression
Automatic Image Compression Like Whatsapp
const result = await Image.compress('file://path_of_file/image.jpg', {
compressionMethod: 'auto',
});
Manual Image Compression
const result = await Image.compress('file://path_of_file/image.jpg', {
maxWidth: 1000,
quality: 0.8,
});
III. Compress the Image
import { View, Image, Button } from 'react-native';
import RNFS from 'react-native-fs';
import ImageCompressor from 'react-native-image-compressor';
const CompressedImage = () => {
const [compressedImage, setCompressedImage] = React.useState(null);
const handleCompress = () => {
var result = await Image.compress('file://path_of_file/image.jpg', {
maxWidth: 1000,
quality: 0.8,
});
setCompressedImage(result);
};
return (
<View>
<Image source={compressedImage} style={{ width: 200, height: 200 }} />
<Button title="Compress" onPress={handleCompress} />
</View>
);
};
export default CompressedImage;
IV. Save the Compressed Image
To save a compressed image in a React Native app, you can use the react-native-fs library to write the compressed image data to a file. Here's an example code snippet:
import { View, Image, Button } from 'react-native';
import RNFS from 'react-native-fs';
import ImageCompressor from 'react-native-image-compressor';
const SaveCompressedImage = () => {
const [compressedImage, setCompressedImage] = React.useState(null);
const handleCompress = () => {
var result = await Image.compress('file://path_of_file/image.jpg', {
maxWidth: 1000,
quality: 0.8,
});
setCompressedImage(result);
};
const handleSave = async () => {
const path = RNFS.DocumentDirectoryPath + '/compressedImage.jpg';
await RNFS.writeFile(path, compressedImage, 'base64');
};
return (
<View>
<Image source={compressedImage} style={{ width: 200, height: 200 }} />
<Button title="Compress" onPress={handleCompress} />
<Button title="Save" onPress={handleSave} />
</View>
);
};
export default SaveCompressedImage;
In this example, the ImageCompressor library is used to compress the image file named "originalImage.jpg" with a quality setting of 0.5, a maximum width and height of 500 pixels, and base64 encoding. The compressed image data is stored in the compressedImage state variable.
To save the compressed image data to a file, the RNFS library is used to write the data to the app's document directory with the filename "compressedImage.jpg". The writeFile method is used to write the data as a base64 string.
The SaveCompressedImage component renders an Image component with the compressed image data as the source. Two buttons are rendered that trigger the handleCompress and handleSave functions respectively when pressed. handleCompress compresses the original image and stores the compressed data in the compressedImage state variable, while handleSave writes the compressed image data to a file in the app's document directory.
V. Display the Compressed Image
To display a compressed image in a React Native app, you can use the Image component and pass in the compressed image file as the source. Here's an example code snippet:
import { View, Image } from 'react-native';
const CompressedImage = () => {
return (
<View>
<Image
source={require('./compressedImage.jpg')}
style={{ width: 200, height: 200 }}
/>
</View>
);
};
export default CompressedImage;
In this example, the compressed image file is named "compressedImage.jpg" and is stored in the same directory as the component file. The require function is used to load the image file as the source for the Image component. The style prop is used to set the width and height of the image to 200 pixels each, but you can adjust these values to fit your specific needs.
VI. Conclusion
In conclusion, the React Native Compressor library provides a convenient and efficient way to compress images in a mobile app. It offers a variety of compression options, allowing developers to balance image quality with file size for optimal performance.
Using the Compressor library can greatly reduce the size of images in an app, which can lead to faster load times, lower bandwidth usage, and improved user experience. It can also help to save storage space on the user's device, which can be especially important for apps with a large number of images.
Overall, React Native Compressor is a useful tool for any developer looking to optimize image performance in their mobile app, and it can help to ensure that the app runs smoothly and efficiently for all users.