Using the React useRef Hook
Learn how to use the React useRef hook to access elements and components in your React app. Understand the useRef API and see practical examples of how to use this powerful React hook.
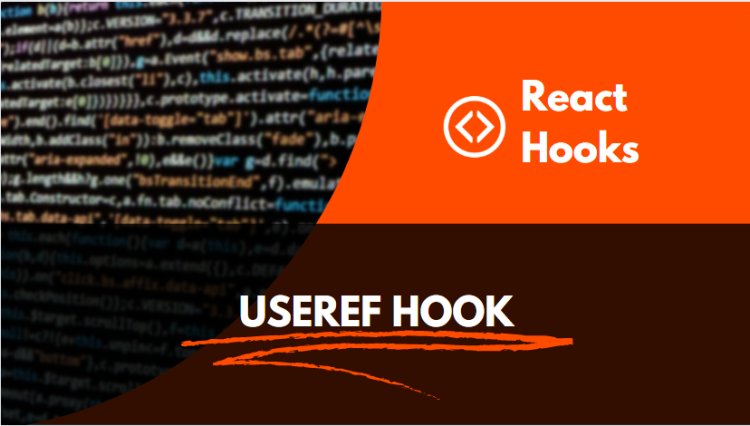
The definition and purpose of the React useRef Hook
The React useRef Hook is used to create a reference to a DOM element or a React component instance. This reference can then be used to access the underlying DOM element or React component instance from within the component.
The useRef Hook is useful for many reasons, but one of its main uses is to maintain a reference to a specific element in the DOM, even if the element has been re-rendered by React. This is because the useRef Hook returns a mutable object that persists for the lifetime of the component. This allows you to access the DOM element directly and update it when necessary, without having to use a callback or a state variable.
Another use of the useRef Hook is to store references to other components and instances. This is especially useful when you have a parent component that needs to communicate with its child components. By storing references to the child components in the useRef Hook, the parent component can access the children directly and update them when necessary.
In short, the useRef Hook is a very useful tool that allows you to create a reference to a DOM element or a React component instance, and access it directly when necessary. It is especially useful for maintaining a reference to a specific element in the DOM, and for communicating with child components from within the parent component.
When to use the React useRef Hook
There are a few occasions where the useRef hook can be useful:
1. When you need a reference to an element that is not a child of the component. For instance, if you need a reference to an element that is outside the render method, you can use the useRef hook.
2. When you need a reference to a component instance. This can be useful if you need to access the component's methods from outside the component.
3. When you need a mutable value. The useRef hook can be used to store a mutable value. This can be useful if you need to store a value that will be updated often.
Since react hooks are a new feature and useref is not a hook, you cannot use it in the same syntax as hooks. However, you can still use useref in React components by declaring a ref variable using the useRef hook and then assigning it to a DOM element. The following example shows how you would use useRef to create a ref variable, assign it to a div element and then access the div's properties:
const MyComponent = () => {
const ref = useRef(null);
return (
<div ref={ref}>
This div can now be accessed via the ref variable.
</div>
);
}
How to use the React useRef Hook
The React useRef hook is a function that allows you to create a reference to a React element or component instance. It's most commonly used to store mutable values across re-renders (for example, a DOM element or a value that doesn't need to trigger a re-render when it changes).
To use the React useRef hook, you must first create a ref instance by calling the useRef hook function. This function takes an initial value as its argument and returns an object containing a current property. This current property should be used when you want to refer to the ref instance.
Once you have your ref instance, you can use it to store any mutable value. For example, let's say you want to store a DOM element that you want to refer back to later. You can use the ref instance to do this by setting the current property of the ref instance to the DOM element:
const myRef = useRef();
// Store a DOM element in the ref instance
myRef.current = document.getElementById('some-element');
// Later, you can access the stored element using the ref instance
const element = myRef.current;
The useRef hook can also be used to store other mutable values, such as a value that doesn't need to trigger a re-render when it changes. For example, let's say you want to store a value that will be used to track the number of times a certain action has been performed. You can use the ref instance to do this by setting the current property of the ref instance to the value you want to track:
const myRef = useRef();
// Store a value in the ref instance
myRef.current = 0;
// Later, you can access the stored value using the ref instance
let count = myRef.current;
// Update the value of the ref instance
myRef.current = count + 1;
By using the React useRef hook, you can create references to React elements or component instances, and store mutable values that don't need to trigger a re-render when they change.
The benefits of using the React useRef Hook
1. Managing Refs: The useRef hook provides a way to store a mutable value in a component's memory. This is useful for storing references to DOM elements, values that need to persist across re-renders, or instance variables that need to be accessed throughout the component.
2. Improving Performance: The useRef hook can also be used to improve performance in React applications. By using the useRef hook instead of creating instance variables, the values stored in the ref will not be recreated on every render, allowing for more efficient code.
3. Creating Timers: The useRef hook can be used to create timers that persist across re-renders. This is useful for creating animations, or for creating a timer that needs to remain running even when the component is re-rendered.
The drawbacks of using the React useRef Hook
1. It can be difficult to keep track of the data stored in the ref object.
2. If not used correctly, useRef can lead to memory leaks.
3. It's not always obvious what kind of data should be stored in the ref object.
4. It can make code more difficult to read and debug.
5. It can be difficult to track changes to the ref object in lifecycle methods.
Example UseRef
import React, { useRef } from 'react';
function App() {
// Create a ref object
const myRef = useRef();
const handleClick = () => {
// Access the ref object's `current` property
myRef.current.style.backgroundColor = 'yellow';
};
return (
<div ref={myRef}>
<button onClick={handleClick}>Click me!</button>
</div>
);
}
export default App;