Use Memo in React Js With Example Code
A memo is a React component that is used to remember the last rendered value of a component. When a value changes, the memo will re-render the component with the new value.
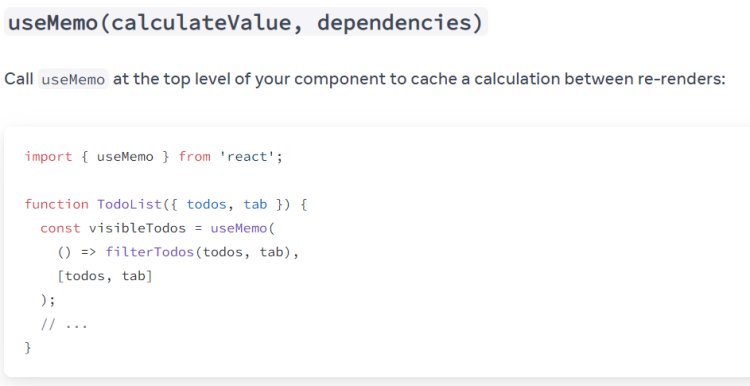
In React, the useMemo
hook is used to optimize the performance of a component by caching the result of a expensive computation. It takes two arguments: a function that performs the computation and an array of dependencies. The hook will only re-run the computation if one of the dependencies has changed.
Here is an example of how you might use the useMemo
hook in a component:
import { useMemo } from 'react';
function MyComponent({ data }) {
const computedValue = useMemo(() => {
// Expensive computation here
return expensiveFunction(data);
}, [data]);return <div>{computedValue}</div>;
}
In this example, the component has a prop called data
and uses the useMemo
hook to perform an expensive computation on it. The hook takes a function that performs the computation and an array of dependencies, in this case just the data
prop. The hook will only re-run the computation if the data
prop changes.
Another way to use useMemo is to return a memoized version of a component, so that the component only re-renders if its props have changed.
import { memo } from 'react';
const MyComponent = memo(function MyComponent({ data }) {
// component logic here
});
In this way, useMemo and memo are similar in that they both help improve the performance of a component by avoiding unnecessary re-renders.
If you're like me, you're constantly looking for ways to optimize your code and make it more efficient. One way to do this is to use memoization in React.
What is memoization? Memoization is a technique for caching and reusing values that have already been calculated. This can be especially useful in React because it can help us avoid re-rendering components unnecessarily.
There are a few different ways to use memoization in React, but one of the most common is to use the React.memo() higher-order component. This HOC will take a component and (optionally) a comparison function as arguments, and return a memoized version of the component.
The comparison function is used to determine whether the component should be re-rendered. If the comparison function returns true, then the component will be re-rendered. If it returns false, then the memoized component will be used instead.
In most cases, you'll want to use the default comparison function, which shallowly compares the props of the two components. However, there are cases where you may want to provide your own comparison function. For example, if your component has a very complex props object, you may want to provide a comparison function that only compares the parts of the props that are relevant to the component.
Once you have a memoized component, you can use it just like you would any other component. The only difference is that it will be much more efficient!
So if you're looking to improve the performance of your React application, consider using memoization. It's a simple technique that can have a big impact.