How to Use Reducer in React to Create Complex Components
Learn how to use reducer in React to create complex components and manage state efficiently. Discover the benefits of using a reducer to create powerful applications that are easier to maintain.
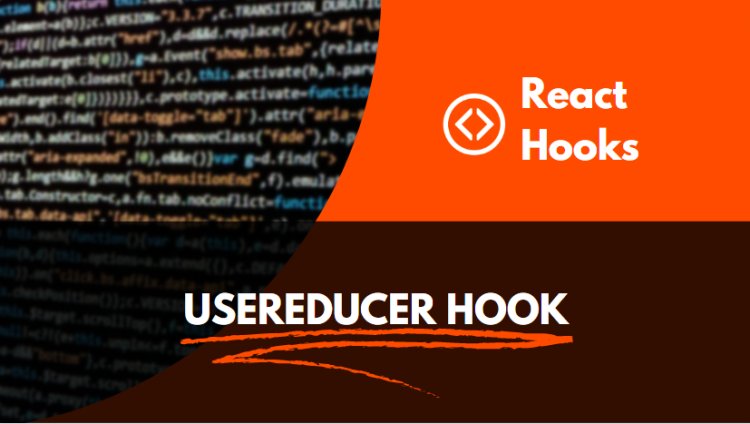
What is a reducer?
A reducer hook is a function that is invoked when a reducer is called. It is used to modify the state before it is passed to the reducer.
A reducer hook is a function that is used to update the state of a React application. It is a way to handle the data within an application, allowing the user to modify the state of the application in a controlled manner. It is typically used in combination with the useState hook, which allows the user to update the state of the application in a more direct manner. The reducer hook is useful for complex updates which require more than one set of parameters to be changed. It is also useful for managing asynchronous actions, such as network requests, within the application.
What are the benefits of using a reducer?
1. Improved code readability: Using a reducer hook allows you to keep the logic for updating your application state separate from the components that use it, making your code more organized and easier to read.
2. Reusability: A reducer hook can be used across multiple components, reducing the need to write duplicate logic in each of them.
3. Easier to debug: With the logic for updating state separated from the components which use it, debugging any issues with the state is much simpler.
4. Controlled mutations: Reducer hooks force you to use a predictable and consistent pattern for updating state, which helps to ensure that state mutations are kept in check.
How to create a simple reducer?
// Reducer hook
const initialState = {
count: 0
};
const reducer = (state, action) => {
switch (action.type) {
case "increment":
return {
count: state.count + 1
};
case "decrement":
return {
count: state.count - 1
};
default:
return state;
}
};
export const useReducer = (reducer, initialState) => {
const [state, dispatch] = useState(initialState);
const dispatchReducer = action => {
dispatch(reducer(state, action));
};
return [state, dispatchReducer];
};
How to use a reducer to create complex components?
If you're looking to create complex components in React, you can do so by using a reducer hook. A reducer hook is a function that takes in two parameters: the current state of the component, and an action to be performed. The reducer hook then returns the new state of the component.
In order to use a reducer hook, you first need to create a new file in your project. Then, import the useReducer hook from React. Next, create a reducer function. This function will take in the current state of the component and an action, and will return the new state.
Finally, use the useReducer hook in your component. This will give you access to the current state of the component and the dispatch function, which you can use to dispatch actions to the reducer function.
With the useReducer hook, you can easily create complex components in React. By dispatching actions to the reducer function, you can update the state of the component and make it do anything you want. Try it out today and see how it can help you create amazing React components.
What are some of the best practices for using a reducer?
1. Use reducer hooks for state that is not derived from props.
2. Keep the reducer hook simple, only contain the logic necessary to modify the state.
3. Split complex logic into separate reducer hooks.
4. Make sure the reducer hook is pure, meaning it does not cause any side effects.
5. Use multiple useReducer hooks for different pieces of state.
6. Leverage the useReducer hook to create derived state.
7. Try to keep the useReducer hook as small as possible.
8. Use useMemo to memoize the values returned from the useReducer hook.
9. Avoid using the useReducer hook for local component state.
10. Leverage the useReducer hook for global state management.
usereducer example program
import React, { useReducer } from 'react';
// Reducer
const reducer = (state, action) => {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
};
// Component
const Counter = () => {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</>
);
};
export default Counter;