Learn How to Use Navigator React Native
Get a step-by-step guide on implementing Navigator React Native in your app. How to Use React Native Navigation
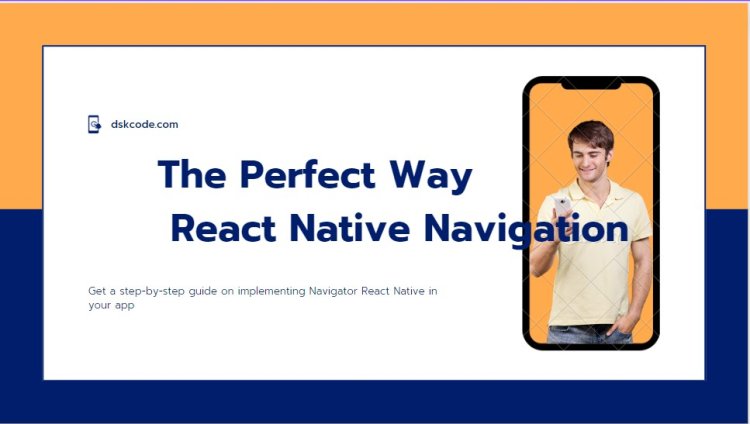
What is React Native Navigation?
React Native Navigation is a library that enables you to implement navigation functionality in your React Native app, utilizing a wide range of navigators offered by the library. The React Native community actively manages RN Navigations, which is based on a similar library for web-based React apps.
With React Native Navigation, you can incorporate various navigation patterns like stack navigator, tab navigator, or drawer navigator, catering to diverse user needs. Its declarative API facilitates simple configuration of navigation options, such as defining the header title or adding buttons, for every screen in your app.
Thanks to its performance, flexibility, and user-friendly approach, React Native Navigation remains a top pick for navigation in RN apps.
Integrating React Native Navigation
Install React Native Navigation dependencies:
Install peer dependencies:
Link dependencies:
Wrap the root component with the NavigationContainer:
function App() {
return (
<NavigationContainer>
{/* Rest of your app */}
</NavigationContainer>
);
}
Create a stack navigator:
const Stack = createStackNavigator();
function MyStack() {
return (
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
);
}
Render the navigator:
function App() {
return (
<NavigationContainer>
<MyStack />
</NavigationContainer>
);
}
Navigate between screens using the navigation prop:
return (
<View>
<Button
title="Go to Details"
onPress={() => navigation.navigate('Details')}
/>
</View>
);
}
Advantages of React Native Navigation
- Performance: RN Navigation is optimized for performance, using native platform-specific components to ensure smooth transitions between screens and efficient memory usage.
- Cross-platform compatibility: RN Navigation works seamlessly on both iOS and Android, allowing developers to create consistent navigation patterns and user experiences across platforms.
- Customizability: RN Navigation provides a flexible API for customizing navigation options and creating unique navigation patterns to fit the needs of your app.
- Easy to use:The declarative API and straightforward setup process make RN Navigation accessible to developers of all skill levels, allowing them to quickly add navigation functionality to their app.
- Active community:RN Navigation is actively maintained by a large community of developers, ensuring that it stays up-to-date with the latest features and bug fixes.
Navigation Lifecycle
For the purpose of navigating through screens in a RN app, the React Navigation library provides a comprehensive solution. Specifically, React Navigation offers a variety of navigators, including stack navigator, tab navigator, and drawer navigator, that can be utilized to create various navigation patterns.
One of the primary challenges of navigating from one screen to another is moving between screens and passing data between them. React Navigation offers the navigate method to move from one screen to another. It handles the underlying logic of how those transitions are executed, such as how the screens are mounted and unmounted, and how to go back.
The navigation process can be thought of as a stack, with screens being pushed and popped from the stack. React Navigation provides various actions, including Replace, Push, Pop, and Pop to top, to manipulate the stack.
To suit different use cases, one can utilize various actions. For instance, to navigate to a new screen and place it on top of the current one, the "Push" action can be used, whereas the "Pop" action can remove one or more screens from the stack.
In our app, we will be using the dispatch to move to the next screen and pass data with it. React Navigation is built on top of RN Navigations, a similar library for web-based React apps, and is actively maintained by the RN community. Its performance, flexibility, and ease of use make it a popular choice for navigation in RN apps.
Summary of React Native Navigation
The Navigator react native library enhances functionality in RN applications by offering a range of navigators that can create various navigation patterns. "In addition, it provides a user-friendly API for configuring navigation options for each individual screen."
RN Navigation boasts several benefits, including optimized performance, compatibility across multiple platforms, customizability, ease of use, and a thriving community of developers.
In conclusion, developers widely adopt and consider RN Navigation as a powerful solution for implementing navigation in React Native applications. Empowering them to provide their users with smooth and streamlined navigation experiences.