A Comprehensive Guide to Using Context and State in Your App
This comprehensive guide explains the basics of React Hooks and how to use them to manage state and context in your React applications. Learn how to access and manipulate state, use custom hooks, and take advantage of the Context API to get the most out of your React components.
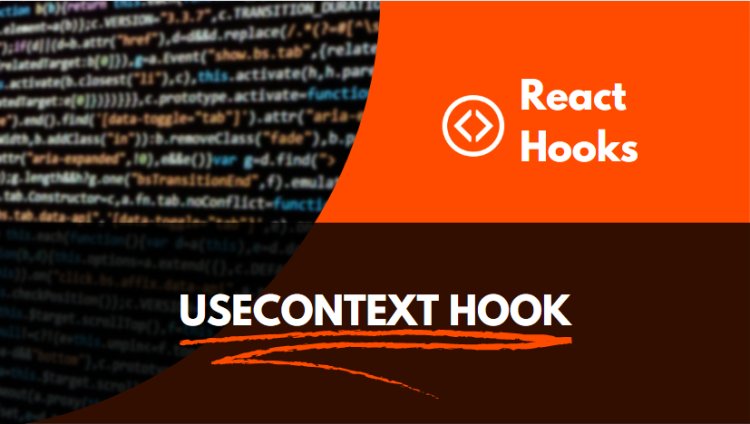
Introduction: what are context and state, and why are they important?
Context and state are two important concepts in computer science that are often used together. Context refers to the set of circumstances or factors that surround a particular event, situation, or thing. State, on the other hand, refers to the current condition or situation of something.
Context and state are important because they can help provide meaning to events and situations. For example, if you know the context of a situation, you may be able to better understand why something happened. Similarly, if you know the state of something, you may be able to better predict what will happen next.
Context and state are also important because they can help you make decisions. For example, if you are considering whether to do something, the context of the situation can help you decide whether it is the right thing to do. Similarly, if you are considering whether to change the state of something, the current state can help you decide whether it is the right thing to do.
The different types of context and state.
There are three different types of context in React: 'parent,' 'children,' and 'grandchildren.' The different types of context dictate how information is passed between React components.
'Parent' context is the simplest form of context and is used when information only needs to be passed from a parent component to its immediate children. 'Children' context is used when information needs to be passed from a parent component to its grandchildren, or from a grandparent component to its grandchildren. 'Grandchildren' context is used when information needs to be passed between a grandparent and its grandchild.
React also has a 'state' object that is used to store information about the component's current state. This state object can be used by any component in the application.
How to use context and state in your app
If you're developing an app that needs to keep track of data or state, you should use Context and State. Context provides a way to pass data through the component tree without having to pass props down manually at every level. State is used to store and manage data within a component.
To use Context and State in your app, you first need to create a context file. This file will export a React Context object and a Provider component. The Provider component is used to provide the Context object to the components in your app.
Next, you need to create a state file. This file will export a React State object. The State object will hold the data that you want to manage within your app.
To use Context and State in your app, you need to import the Context and State objects into your components. You can then use the Context object to pass data down the component tree and the State object to store and manage data within the component.
This example program uses the useContext hook to create a context and access it in a component.
import React, { createContext, useContext } from 'react';
// Create a context
const MyContext = createContext();
// Create a component that provides the context
const MyProvider = ({ children }) => {
const value = {
name: 'John',
age: 32
}
return (
<MyContext.Provider value={value}>
{children}
</MyContext.Provider>
)
}
// Create a component that uses the context
const MyComponent = () => {
const context = useContext(MyContext);
return (
<div>
<p>Name: {context.name}</p>
<p>Age: {context.age}</p>
</div>
)
}
// Render the components
const App = () => {
return (
<MyProvider>
<MyComponent />
</MyProvider>
)
}
export default App;
The benefits of using context and state in your app
When it comes to creating an app, context and state are two of the most important considerations. By understanding and utilizing both of these concepts, you can create a more user-friendly and efficient app.
Context refers to the environment in which an action takes place. It helps to provide meaning to the action and can influence how it is interpreted. State, on the other hand, is the current status of an object. Together, context and state can be used to create a more seamless user experience.
For example, consider a to-do list app. The app might use context to help the user understand why a particular task is important. The app might also use state to track the progress of the task and whether it has been completed.
By utilizing both context and state, you can create an app that is more user-friendly and efficient.
The drawbacks of using context and state in your app
When using context and state in your app, there are a few potential drawbacks to be aware of. First, if your app is complex, managing all of the different contexts and states can be difficult. Second, if you make changes to the context or state, it can be difficult to track those changes and ensure that they are reflected in the app correctly. Finally, if you use too much context or state in your app, it can start to feel cluttered and overwhelming.