Javascript Interview Questions for Freshers
Prepare for your JavaScript interview with these essential interview questions that every fresher should know.
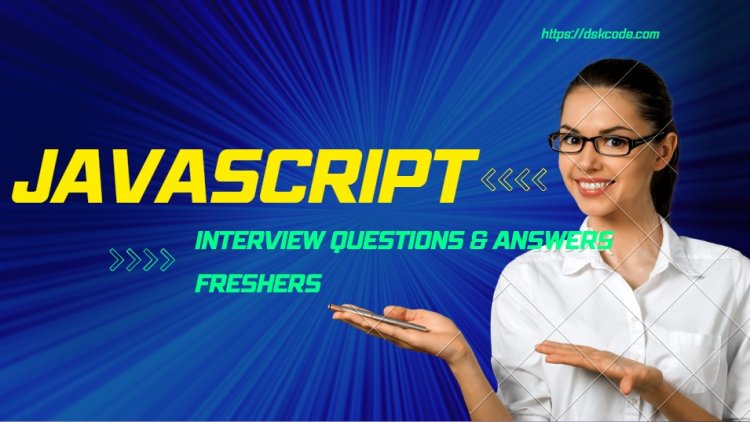
What is JavaScript?
JavaScript is a high-level, dynamic, and interpreted programming language that is primarily used to create interactive web pages and dynamic web applications. It is a client-side scripting language that runs on the user's browser.
<!DOCTYPE html> <html> <head> <title>JavaScript Example</title> </head> <body> <script> // alert("Hello, World!"); </script> </body> </html>
This example demonstrates how to use JavaScript to display a message box with the text "Hello, World!" when the web page loads.
What are the differences between JavaScript and Java?
Java is a statically-typed, compiled programming language that is used primarily for building large-scale enterprise applications. JavaScript is a dynamically-typed, interpreted programming language that is used primarily for building client-side web applications.
What are the data types in JavaScript?
JavaScript has six primitive data types: string, number, boolean, null, undefined, and symbol. It also has one non-primitive data type: object.
let str = "Hello"; let num = 42; let bool = true; let n = null; let undef; let sym = Symbol(); let obj = {name: "John", age: 25};
- Number: represents both integer and floating-point numbers
- String: represents a sequence of characters enclosed in single or double quotes.
- Boolean: represents a logical entity that can have two values: true or false.
- Null: represents a deliberate non-value, assigned using the keyword null.
- Undefined: represents an uninitialized value, assigned automatically when a variable is declared but not assigned a value.
- Symbol: represents a unique identifier that can be used as a property name.
- Object: represents a collection of properties and methods, and can contain values of any of the other data types.
What is a variable in JavaScript?
A variable in JavaScript is a container that holds a value. Variables can be declared using the keywords var, let, or const.
The var keyword was traditionally used to declare variables, but starting from ES6 (ECMAScript 2015), let and const were introduced as block-scoped alternatives to var.
It's worth noting that let and const are block-scoped, meaning that they are only accessible within the block in which they are defined. var, on the other hand, is function-scoped, meaning that it is accessible within the entire function in which it is defined.
var x = 5; let y = 10; const z = 15;
What is a function in JavaScript?
A function in JavaScript is a block of code that performs a specific task. Functions are used to perform repetitive tasks, to group code that performs a specific task, and to make code more modular and easier to read.
function add(a, b) { return a + b; }
This function takes two arguments, a and b, and returns their sum.
What is an array in JavaScript?
An array in JavaScript is a collection of elements of any data type. Arrays can be declared using square brackets [], and elements can be accessed using their index.
An array in JavaScript is an object that stores multiple values in a single variable. Arrays are indexed and can contain any type of data, including strings, numbers, and other objects. They are commonly used to store collections of data, such as a list of items or a set of related variables. Arrays can also be used as a way to organize data and enable certain operations to be performed on the data.
let arr = [1, "two", true]; console.log(arr[0]); // Output: 1
What is an object in JavaScript?
An object in JavaScript is a collection of key-value pairs. Objects can be declared using curly braces {}, and values can be accessed using their key.
An object in JavaScript is a data type which is composed of a collection of key/value pairs. Objects can represent real-world objects, such as a person, or they can represent abstract objects, such as a set of numbers or a stack of data. Objects can contain other objects, functions, and primitive data types.
let person = { name: "John", age: 25, gender: "male" }; console.log(person.name); // Output: John
What is a loop in JavaScript?
A loop in JavaScript is a way to repeat a block of code multiple times. There are two main types of loops in JavaScript: for and while.
A loop is a sequence of instructions that is continually repeated until a certain condition is met. In JavaScript, there are several kinds of loops such as for, while, do-while and for-in loops. The for loop is the most commonly used loop in JavaScript, and it is used to iterate over an array or object. It is typically used to execute a set of instructions a specified number of times. The while loop is used to execute a set of instructions as long as a certain condition is true. The do-while loop is similar to the while loop, but it executes the instructions at least once before checking the condition. The for-in loop is used to iterate over the properties of an object.
// Using a for loop for (let i = 0; i < 5; i++) { console.log(i); } // Using a while loop let j = 0; while (j < 5) { console.log(j); j++; }
What is an if-else statement in JavaScript?
An if-else statement in JavaScript is a way to execute code conditionally. If the condition is true, the code inside the if block is executed, otherwise the code inside the else block is executed.
let x = 5; if (x > 10) { console.log("x is greater than 10"); } else { console.log("x is less than or equal to 10"); }
What are the types of operators in JavaScript?
JavaScript has several types of operators, including arithmetic, assignment, comparison, logical, and bitwise operators.
// Arithmetic operators let x = 10; let y = 5; console.log(x + y); // Output: 15 console.log(x - y); // Output: 5 console.log(x * y); // Output: 50 console.log(x / y); // Output: 2 // Assignment operators let z = 15; z += 5; // Equivalent to z = z + 5 console.log(z); // Output: 20 // Comparison operators let a = 10; let b = 5; console.log(a > b); // Output: true console.log(a < b); // Output: false console.log(a == b); // Output: false console.log(a != b); // Output: true // Logical operators let c = 20; let d = 25; console.log(c > 10 && d < 30); // Output: true console.log(c < 10 || d > 30); // Output: false // Bitwise operators let e = 5; // Binary 101 let f = 3; // Binary 011 console.log(e & f); // Output: 1 (Binary 001) console.log(e | f); // Output: 7 (Binary 111)
What is the this keyword in JavaScript?
The this keyword in JavaScript refers to the object that the function belongs to, or the object that is being operated on. It is used to access the object's properties and methods from within the function.
let person = { name: "John", age: 25, sayHello: function() { console.log("Hello, my name is " + this.name); } }; person.sayHello(); // Output: Hello, my name is John
What is the difference between var, let, and const in JavaScript?
var is used to declare variables that have global or function scope. let is used to declare variables that have block scope, and const is used to declare variables that cannot be reassigned.
// Using var function foo() { var x = 5; if (true) { var x = 10; } console.log(x); // Output: 10 } // Using let function bar() { let y = 5; if (true) { let y = 10; } console.log(y); // Output: 5 } // Using const const z = 5; z = 10; // This will throw an error
What is a promise in JavaScript?
A promise in JavaScript is an object that represents the eventual completion or failure of an asynchronous operation. Promises provide a way to write asynchronous code that is easier to read and understand.
function getData() { return new Promise(function(resolve, reject) { // Perform asynchronous operation here // If the operation is successful, call resolve() with the data // If the operation fails, call reject() with an error }); } getData().then(function(data) { console.log(data); }).catch(function(error) { console.log(error); });
What is event bubbling in JavaScript?
Event bubbling in JavaScript is a phenomenon where when an event is triggered on an element, the event is first handled by the element, and then propagated to its parent elements in the DOM tree. This continues until the event reaches the top-most element, such as the document object. In this example, if the button is clicked, both the button and the div will log messages to the console because the event bubbles up from the button to the div.
<div onclick="console.log('Clicked div');"> <button onclick="console.log('Clicked button');">Click me</button> </div>
What is a closure in JavaScript?
A closure in JavaScript is a function that has access to variables in its outer function, even after the outer function has returned. Closures are created when a function is defined inside another function, and the inner function references variables from the outer function.
function outer() { let x = 5; function inner() { console.log(x); } return inner; } let func = outer(); func(); // Output: 5
In this example, outer() returns the inner() function, which still has access to the x variable from outer() even after outer() has returned. When func() is called, it logs the value of x to the console.