JavaScript Coding Questions and Answers: An Essential Guide
A comprehensive guide to JavaScript coding questions and answers, essential for mastering the language. Learn key concepts, tips, and strategies for tackling coding problems.
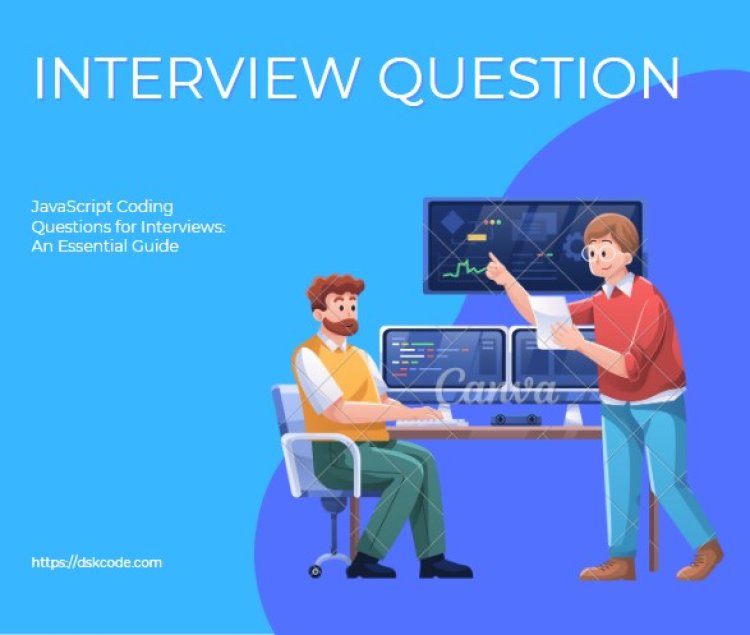
Coding Round Tips and Tricks:
1. Understand the problem: Read the problem statement carefully and try to understand the problem. Ask questions if needed to get a better understanding of the problem.
2. Break it down: Break the problem into smaller sub-problems and try to solve them one by one.
3. Brainstorm: Think of different approaches to solve the problem and choose the best approach that suits your needs.
4. Write pseudocode: Write down the pseudocode for the problem. This helps in visualizing the problem better and keeps you from getting stuck in the middle.
5. Test your code: Make sure to test your code for different inputs and corner cases.
6. Optimize your code: Try to optimize your code to make it as efficient as possible.
7. Document your code: Write comments in your code to make it easier for others to understand.
Reverse a String: Write a function that takes a string input and returns the string reversed.
Explanation: The function uses the split('') method to convert the string into an array of characters, then uses the reverse() method to reverse the order of the characters, and finally uses the join('') method to convert the array back into a string in reversed order.
return str.split('').reverse().join('');
}
Find the Longest Word: Write a function that takes a sentence as input and returns the longest word in the sentence.
Explanation: The function uses the split(' ') method to split the sentence into an array of words, then iterates through the words using a for...of loop, keeping track of the longest word found so far. It returns the longest word after the loop completes.
const words = sentence.split(' ');
let longestWord = '';
for (const word of words) {
if (word.length > longestWord.length) {
longestWord = word;
}
}
return longestWord;
}
Palindrome Check: Write a function that takes a string input and checks if it's a palindrome (reads the same backward as forward).
Explanation: The function uses the split('') method to convert the string into an array of characters, then uses the reverse() method to reverse the order of the characters, and finally uses the join('') method to convert the array back into a string in reversed order. It then compares the original string with the reversed string to check if they are the same.
const reversed = str.split('').reverse().join('');
return str === reversed;
}
Fibonacci Series: Write a function that generates the Fibonacci series up to a given number.
Explanation: The function uses a loop to generate the Fibonacci series up to the given number. It starts with an array containing the initial two numbers (0 and 1), and then iterates from the third position in the array, adding the two previous numbers to generate the next number in the series.
const series = [0, 1];
for (let i = 2; i < n; i++) {
series[i] = series[i - 1] + series[i - 2];
}
return series;
}
Array Sum: Write a function that takes an array of numbers and returns the sum of all the numbers in the array.
Explanation: The function uses a loop to iterate through the array and adds each number to a running total. It then returns the total sum after the loop completes.
let sum = 0;
for (const num of arr) {
sum += num;
}
return sum;
}
Find Duplicates: Write a function that takes an array of numbers and returns an array containing any duplicates.
Explanation: The function uses an object to keep track of the count of each number in the array. It then iterates through the array, checking if the count of a number is greater than 1, which indicates a duplicate. If found, the number is added to the duplicates array.
const duplicates = [];
const numCount = {};
for (const num of arr) {
if (!numCount[num]) {
numCount[num] = 1;
} else if(numCount[num] === 1) {
duplicates.push(num);
}
}
return duplicates;
}
Two Sum: Given an array of numbers and a target sum, write a function that finds two numbers in the array whose sum equals the target sum and returns their indices.
Explanation: The function uses a hash map to keep track of the numbers in the array as it iterates through them. For each number, it calculates the complement (target sum minus the current number) and checks if the complement exists in the hash map. If found, it returns the indices of the current number and its complement.
const numMap = new Map();
for (let i = 0; i < arr.length; i++) {
const complement = targetSum - arr[i];
if (numMap.has(complement)) {
return [numMap.get(complement), i];
}
numMap.set(arr[i], i);
}
return [];
}
Object Manipulation: Given an array of objects, write a function that returns a new array containing only the objects that meet a certain condition.
Explanation: The function uses the filter() method to create a new array containing only the objects that satisfy the given condition. The condition is defined as a function that takes an object as input and returns a boolean value.
return arr.filter(condition);
}
Object Merging: Given two objects, write a function that merges them into a single object.
Explanation: The function uses the Object.assign() method to merge the properties of the two objects into a new object. It returns the merged object.
return Object.assign({}, obj1, obj2);
}
Object Sorting: Given an array of objects, write a function that sorts them based on a given property in ascending or descending order.
Explanation: The function uses the sort() method to sort the array of objects based on the given property. The sorting order (ascending or descending) is determined by a parameter. The function uses a custom comparison function to compare the values of the given property in the objects.
return arr.sort((a, b) => {
if (order === 'asc') {
return a[property] - b[property];
} else {
return b[property] - a[property];
}
});
}