UseSelector A Guide to React Redux Hooks
Improve productivity and simplify state management with useSelector
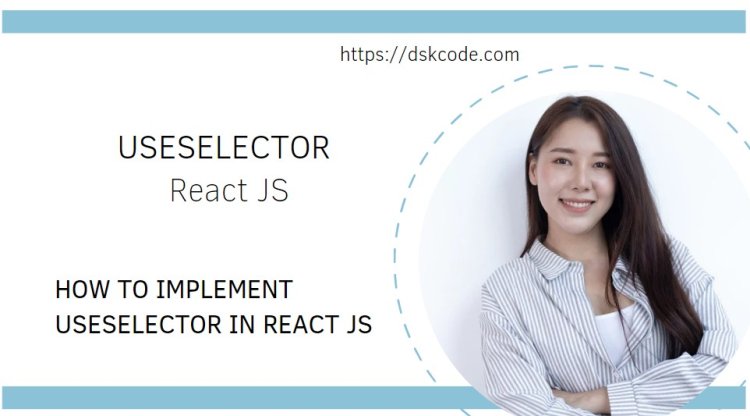
USESELECTOR AND USEDISPATCH IN REACT REDUX
Using useSelector and useDispatch allows for an alternative to the connect() higher-order component. useSelector can replace map state to props by taking in a function argument that specifies the desired part of the state. On the other hand, useDispatch can replace map dispatch to props. To utilize useDispatch, it can be invoked and stored to a variable such as dispatch. This dispatch variable can then work with allActions imported from the actions folder.
Getting Started
Install necessary dependencies
npm install react-redux
what is redux?
Redux is a popular open-source JavaScript library that provides a centralized approach to managing application state. It is frequently used in conjunction with React JS or Angular to build user interfaces. In Redux, the state of the application is held in a store, and components can access relevant state from the store as needed. By using Redux, developers can ensure that state management is predictable and centralized, which makes applications easier to maintain, debug, and test.
what is react-redux?
React-Redux is a library that facilitates the integration of Redux with React JS, enabling the use of a global state management system. It offers bindings that enable React components to interact with the Redux store and dispatch actions. This allows developers to take a cohesive approach to state management in their React applications, improving efficiency and consistency.
Create Redux store
// store.js
import { createStore } from 'redux';
const initialState = { counter: 0 };
function reducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return { ...state, counter: state.counter + 1 };
case 'DECREMENT':
return { ...state, counter: state.counter - 1 };
default:
return state;
}
}
const store = createStore(reducer);
export default store;
What is Redux Store?
Redux is a widely used state management library for JavaScript applications, typically in conjunction with React. However, it is also possible to use it with other view libraries. The Redux store is a central object that holds the state of the application. As the sole source of truth for the application's state, the store provides a reliable API for reading and modifying the state. Additionally, Redux enforces a strict unidirectional data flow, which ensures that the state remains consistent and predictable throughout the application.
Implement Redux
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import store from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Why implementing Redux in Root File?
- Wrap your entire app with a Provider component from react-redux, which provides access to the Redux store to all components in the app.
- Set up any middleware or enhancers that you want to use with your store, such as redux-thunk for handling asynchronous actions, or redux-devtools-extension for debugging your store in the browser.
- Configure the initial state of your store and set up any necessary reducers or actions.
- Easily manage any changes or updates to your store, such as adding new reducers or middleware, by making changes in a single centralized location.
Implementing Redux in the root file also helps to improve the overall performance and efficiency of your app. By creating a single global store instance, you can avoid duplicating state across multiple components, and ensure that all components have access to the same up-to-date data.
Selector function
Create a selector function to extract the counter state from the store:
// selectors.js
export const selectCounter = state => state.counter;
Display Data
Create a React component that uses useSelector to access the counter value and display it
// Counter.js
import React from 'react';
import { useSelector } from 'react-redux';
import { selectCounter } from './selectors';
function Counter() {
const counter = useSelector(selectCounter);
return (
<div>
<p>Count: {counter}</p>
</div>
);
}
export default Counter;
Render the Counter component in your app
// App.js
import React from 'react';
import Counter from './Counter';
function App() {
return (
<div>
<Counter />
</div>
);
}
export default App;
benefits of useSelector
- Simplified code:
With useSelector, you don't need to write a separate mapStateToProps function and connect your component to the store using connect. Instead, you can define a selector function inline and use it directly in your component.
- Efficient updates:
useSelector uses the strictEqual comparison algorithm to compare the selected data with its previous value, which means that your component will only re-render when the selected data has actually changed.
- Memoization:
useSelector automatically memoizes the selected data, which means that if the dependencies of your selector function haven't changed, it will return the previously computed value instead of recomputing it.
- Easy testing:
Since useSelector is just a regular JavaScript function, you can easily test it in isolation without needing to render your entire component hierarchy or mock the Redux store.
In addition to these benefits, useSelector also supports more advanced features like selecting data from nested slices of the store, composing selectors, and using selectors with the useMemo hook to further optimize performance.
Overall, useSelector is a powerful tool for working with Redux in React, and its simplicity and efficiency make it a great choice for building scalable and maintainable applications.