Top JavaScript Interview Question and Answer
If you're looking for top JavaScript interview questions and answers, then you've come to the right place. In this article, we'll share with you some of the most commonly asked questions in JavaScript interviews, along with their answers.
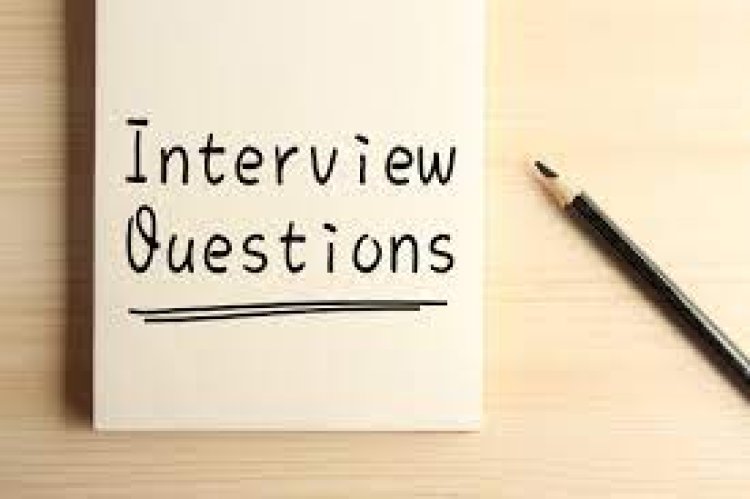
What is the difference between == and ===?
The == operator is an equality operator, which means it checks if the two operands are equal in value. The === operator is a strict equality operator, which means it checks if the two operands are equal in value and type.
What is the difference between null and undefined?
Null and undefined both represent the absence of a value, but they are different types of values. Null is a value of the null type, while undefined is a value of the undefined type. This means that you can test for the absence of a value with the === operator, but you can't test for the type of value with the typeof operator.
Null is often used to represent the absence of a value when no other value makes sense. For example, you might use null to represent the fact that a user hasn't selected a value from a drop-down list.
Undefined is often used to represent the absence of a value when the value is known but not yet assigned. For example, if you declare a variable but don't assign it a value, the variable will have the value undefined.
What is a closure?
A closure is used to create a function that will have access to variables that are not in the global scope. This is useful when you want to create a function that will work with data that is not global, such as data passed into the function as an argument.
The following example creates a function that takes an argument and returns a function that increments the argument. The inner function has access to the variable i that is defined in the outer function.
function makeAdder(i) {
return function(j) {
return i + j;
};
}
var add5 = makeAdder(5);
var add10 = makeAdder(10);
console.log(add5(2)); // 7
console.log(add10(2)); // 12
What is the difference between a function and a method?
What is the difference between an object and an array?
What is the difference between a class and an interface?
What is the difference between a static and an instance method?
An instance method is a method that is associated with an instance of a class. An instance method can be invoked only after creating an instance of the class.