React Native Flatlist Typescript
Explore the recommended best practices for optimizing your usage of React Native Flatlist Typescript in mobile app development.
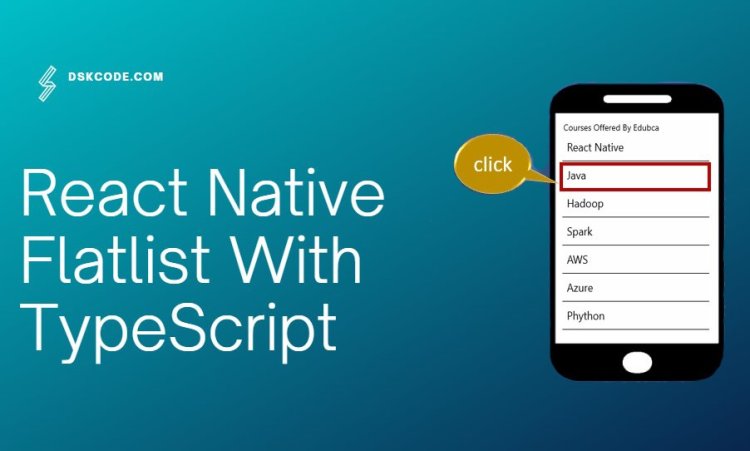
What is FlatList in React Native?
In React Native FlatList is a built-in component that provides an efficient way to render lists of data. It is designed to efficiently handle large lists by rendering only the visible items on the screen, recycling the off-screen items as the user scrolls.
The FlatList component accepts an array of data and a function to render each item in the list. It automatically manages the rendering and re-rendering of the items based on the data changes and user interactions.
Here are some key features and benefits of using FlatList:
- Efficient rendering: FlatList renders only the items that are currently visible on the screen, which improves the performance and memory usage of your app when dealing with large datasets.
- Virtualized scrolling: It efficiently manages the memory and scrolling behavior, allowing for smooth and responsive scrolling even with a large number of items.
- Dynamic data updates: When the underlying data changes, FlatList automatically re-renders the affected items, ensuring that your UI stays up to date with the latest data.
- Built-in item key extraction: It provides a keyExtractor prop to extract unique keys from the data items, which helps optimize the rendering and reordering of the list items.
- Customization and flexibility: FlatList offers various props and callbacks to customize the appearance and behavior of the list, such as item separators, empty list rendering, scroll indicators, and more.
Overall, FlatList simplifies the process of rendering and managing lists of data in React Native applications, providing a performant and efficient solution for displaying scrollable content.
What is the benefit of FlatList in React Native?
The FlatList component in React Native offers several benefits for efficiently rendering lists of data in your applications:
- Memory efficiency: FlatList renders only the visible items on the screen, recycling the off-screen items as the user scrolls. This approach significantly reduces memory usage compared to rendering all items at once, making it suitable for handling large datasets without sacrificing performance.
- Performance optimization: By rendering only the visible items, FlatList ensures smooth scrolling performance, even when dealing with a large number of items. It leverages the concept of virtualization to efficiently manage the memory and re-rendering of items, resulting in a responsive user experience.
- Dynamic updates: When the underlying data changes, such as adding, removing, or updating items, FlatList automatically re-renders the affected items while keeping the rest of the list intact. This dynamic update capability simplifies the process of reflecting changes in your UI without manual intervention.
- Key extraction: FlatList provides a keyExtractor prop that allows you to specify how to extract unique keys from your data items. Properly defined keys optimize the rendering and reordering of the list items, ensuring efficient updates and preventing duplicate key errors.
- Customization options: FlatList offers various props and callbacks to customize the appearance and behavior of the list. You can define item separators, empty list renderings, scroll indicators, and more to adapt the FlatList to your specific design and user experience requirements.
- Accessibility support: FlatList supports accessibility features out of the box, making it easier to create inclusive applications. It ensures that screen readers can navigate the list items and provides accessibility-related props to enhance the user experience for people with disabilities.
FlatList component simplifies the process of rendering and managing lists of data in React Native, providing memory efficiency, performance optimization, dynamic updates, and customization options. These benefits contribute to a smoother and more responsive user interface, especially when dealing with large or dynamically changing datasets.
How to create a React Native FlatList component using TypeScript
Step 1: Set up a new React Native project
Create a new React Native project using the following command:
Step 2: Install dependencies
Navigate into the project directory and install the necessary dependencies
cd FlatListExample
npm install
Step 3: Create a FlatList component
Open the App.tsx file in the project's root directory and replace its content
import { FlatList, StyleSheet, Text, View } from 'react-native';
// Define the item type
type ListItem = {
id: number;
title: string;
};
const App: React.FC = () => {
// Sample data
const data: ListItem[] = [
{ id: 1, title: 'Item 1' },
{ id: 2, title: 'Item 2' },
{ id: 3, title: 'Item 3' },
{ id: 4, title: 'Item 4' },
];
// Render item function
const renderItem = ({ item }: { item: ListItem }) => (
<View style={styles.item}>
<Text style={styles.title}>{item.title}</Text>
</View>
);
return (
<View style={styles.container}>
<FlatList
data={data}
renderItem={renderItem}
keyExtractor={(item) => item.id.toString()}
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
marginTop: 50,
},
item: {
backgroundColor: '#f9c2ff',
padding: 20,
marginVertical: 8,
marginHorizontal: 16,
},
title: {
fontSize: 16,
},
});
export default App;
Step 4: Run the app
Start the React Native development server and run the app on a connected device or emulator using the following command:
(or)
That's it! You should now see a basic React Native app with a FlatList component displaying the sample data.
React Native Flatlist Sample Program that displays a list of countries
import { FlatList, StyleSheet, Text, View, TouchableOpacity } from 'react-native';
// Define the item type
type CountryItem = {
id: number;
name: string;
};
const App: React.FC = () => {
// Sample data
const data: CountryItem[] = [
{ id: 1, name: 'United States' },
{ id: 2, name: 'United Kingdom' },
{ id: 3, name: 'Canada' },
{ id: 4, name: 'Australia' },
];
// State for selected country
const [selectedCountry, setSelectedCountry] = useState
// Render item function
const renderItem = ({ item }: { item: CountryItem }) => (
<TouchableOpacity
style={[styles.item, selectedCountry?.id === item.id && styles.selectedItem]}
onPress={() => setSelectedCountry(item)}
>
<Text style={styles.title}>{item.name}</Text>
</TouchableOpacity>
);
return (
<View style={styles.container}>
<FlatList
data={data}
renderItem={renderItem}
keyExtractor={(item) => item.id.toString()}
ItemSeparatorComponent={() => <View style={styles.separator} />}
/>
{selectedCountry && (
<View style={styles.selectedCountry>
<Text style={styles.selectedCountryText}>Selected Country: {selectedCountry.name}</Text>
</View>
)}
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: 50,
paddingHorizontal: 16,
},
item: {
backgroundColor: '#f9c2ff',
padding: 20,
marginVertical: 8,
borderRadius: 8,
},
selectedItem: {
backgroundColor: '#a6c2ff',
},
title: {
fontSize: 16,
},
separator: {
height: 1,
backgroundColor: '#ccc',
},
selectedCountry: {
marginTop: 20,
alignItems: 'center',
},
selectedCountryText: {
fontSize: 18,
fontWeight: 'bold',
},
});
export default App;
In this example, we have a list of countries stored in the data array. The FlatList component is used to render the list, and each country item is rendered using the renderItem function. The selected country is stored in the selectedCountry state variable, and when a country item is pressed, it becomes the selected country.
The selected country is then displayed below the FlatList component.
The styles defined in the StyleSheet.create function are used to style the components, including the item, selected item, separator, and selected country.
Feel free to customize the data array, add more properties to the CountryItem type, and modify the styling according to your requirements.