Top 25 Node.js Interview Questions and Answers: Ace Your Next Interview with Confidence
Discover the best Node.js Interview Questions and Answers to help you ace your next interview and gain the confidence you need to succeed!
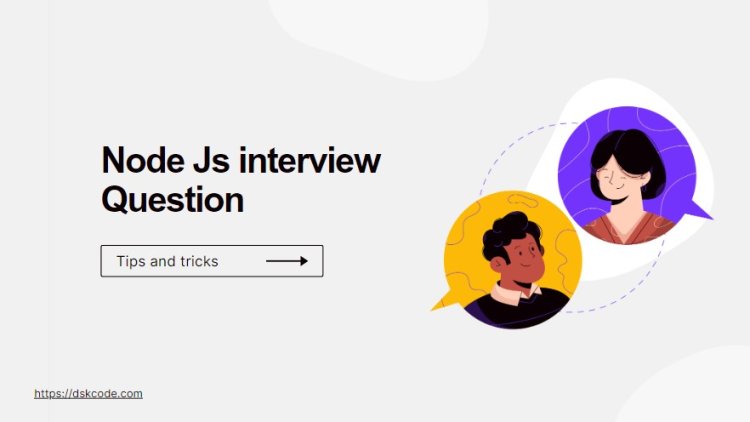
Question: What is Node.js?
Answer: Node.js is a server-side JavaScript runtime environment built on Chrome's V8 JavaScript engine. It allows developers to run JavaScript on the server side, enabling server-side scripting and building scalable network applications.
Question: Explain the event-driven architecture in Node.js.
Answer: Node.js follows an event-driven architecture, where the execution flow is based on events and callbacks. It uses an event loop to handle multiple concurrent connections efficiently. When an event occurs, a callback function is triggered, and the server continues to process other requests without blocking the execution flow.
Question: What is the purpose of the "require" function in Node.js?
Answer:The "require" function in Node.js is used to load modules into a Node.js application. It allows you to reuse code and import functionality from other modules into your application.
Question: What is a callback in Node.js?
Answer:A callback is a function that is passed as an argument to another function and is called when that function completes its execution. In Node.js, callbacks are commonly used for asynchronous operations, such as file I/O or database queries, to handle results or errors.
Question: What is the significance of the "package.json" file in Node.js?
Answer: The "package.json" file is used to define a Node.js application's metadata, including its name, version, dependencies, and scripts. It allows developers to manage their application's dependencies, configuration, and scripts in a centralized manner.
Question: How can you handle errors in Node.js?
Answer:Errors in Node.js can be handled in several ways, such as using try-catch blocks, using error-first callbacks, using promises with .catch(), using event emitters to emit error events, or using error handling middleware in Express.js.
Question: Explain the concept of streams in Node.js.
Answer:Streams in Node.js are used to handle large data sets and perform operations on them in a memory-efficient and scalable manner. Streams allow data to be processed in chunks, rather than loading the entire data into memory, which can be beneficial for processing large files, network data, or data from databases.
Question: What are the core modules in Node.js?
Answer:Node.js has several core modules built into its core library, including "http", "fs", "path", "os", "events", "util", and "stream". These modules provide essential functionality for building server-side applications in Node.js.
Question: What is the difference between "process.nextTick()" and "setImmediate()" in Node.js?
Answer: "process.nextTick()" and "setImmediate()" are both used for scheduling callbacks in the Node.js event loop. However, "process.nextTick()" executes the callback immediately after the current operation completes, before the event loop continues, while "setImmediate()" schedules the callback to be executed in the next iteration of the event loop.
Question: Explain the concept of middleware in Express.js.
Answer:Middleware in Express.js are functions that can process incoming HTTP requests and modify them or perform additional operations before they are handled by the final request handler. Middleware functions can be used for tasks such as authentication, validation, logging, error handling, and more.
Question: What is the purpose of the "module.exports" and "exports" objects in Node.js?
Answer:In Node.js, "module.exports" and "exports" are objects that are used to define the public interface of a module and make it accessible to other modules. "module.exports" is the actual object that is exported as the module, while "exports" is a reference to "module.exports" for convenience.
Question: What is the purpose of the "Buffer" class in Node.js?
Answer:The "Buffer" class in Node.js is used to handle binary data, such as reading from or writing to sockets, working with file streams, or handling data encryption/decryption. It allows you to manipulate binary data in the form of bytes and provides methods for encoding and decoding data in various formats, such as UTF-8, Base64, and hexadecimal.
Question: What is the role of the "cluster" module in Node.js?
Answer:The "cluster" module in Node.js is used to create child processes that run in parallel and share the same server port. It allows you to take advantage of multi-core CPUs and distribute the processing load across multiple CPU cores, thereby improving the performance and scalability of Node.js applications.
Question: How can you handle file uploads in Node.js?
Answer:File uploads in Node.js can be handled using libraries such as "multer" or "formidable", which provide middleware for parsing and processing file uploads from HTTP requests. These libraries allow you to handle file uploads securely, configure file size limits, handle file type validation, and save uploaded files to the server or cloud storage.
Question: Explain the concept of Promises in Node.js.
Answer:Promises in Node.js are used to handle asynchronous operations in a more readable and organized manner. Promises represent a value that may not be available yet, but will be resolved in the future. They allow you to chain multiple asynchronous operations together, handle errors in a centralized way, and improve the overall readability and maintainability of asynchronous code.
Question: What is the purpose of the "vm" module in Node.js?
Answer:The "vm" module in Node.js is used to run JavaScript code within a Node.js application as a separate context. It provides methods for compiling and executing JavaScript code dynamically, sandboxing the execution environment, and managing global objects, thereby allowing you to run untrusted or dynamic code securely.
Question: How can you handle authentication in Node.js applications?
Answer:Authentication in Node.js applications can be implemented using various techniques, such as using passport.js middleware, implementing token-based authentication with JWT (JSON Web Tokens), integrating with OAuth providers, or using custom authentication strategies. Authentication involves verifying user credentials, managing sessions or tokens, and implementing authorization logic to restrict access to protected resources.
Question: Explain the concept of "callback hell" in Node.js and how to avoid it.
Answer:"Callback hell" is a term used to describe the nested and complex structure of callbacks that can occur in Node.js when dealing with multiple asynchronous operations. It can result in unreadable and hard-to-maintain code. To avoid callback hell, you can use techniques such as modularization, using named functions instead of anonymous functions, using promises, or utilizing async/await syntax, which provides a more linear and organized way of handling asynchronous operations.
Question: What is the purpose of the "child_process" module in Node.js?
Answer:The "child_process" module in Node.js is used to create, manage, and communicate with child processes. It allows you to spawn new processes, execute shell commands, communicate with child processes using stdin/stdout/stderr, and handle events related to child processes. This module is useful for tasks such as running external scripts, executing system commands, or creating concurrent processes.
Question: How can you handle caching in Node.js applications?
Answer:Caching in Node.js applications can be implemented using various techniques, such as in-memory caching using objects or maps, using external caching stores like Redis or Memcached, implementing server-side caching with middleware like "express-cache-controller", or using caching libraries like "node-cache" or "memory-cache". Caching can help improve the performance and scalability of applications by storing frequently accessed data in memory for faster retrieval.
Question: What is the difference between "setImmediate()" and "setTimeout()" in Node.js?
Answer: "setImmediate()" and "setTimeout()" are both used to schedule asynchronous code execution in Node.js, but they have some differences. "setImmediate()" is used to execute a callback function after the current event loop iteration, while "setTimeout()" is used to execute a callback function after a specified delay in milliseconds. "setImmediate()" callbacks are executed before "setTimeout()" callbacks with a delay of 0 milliseconds, making them more efficient for immediate execution of asynchronous code.
Question: Explain the concept of "middleware" in Node.js.
Answer:Middleware in Node.js refers to a series of functions that are executed in sequence in the order they are defined in the request-response lifecycle. Middleware functions have access to the request and response objects, and they can modify them, perform actions, or pass control to the next middleware in the chain. Middleware is commonly used for tasks such as handling authentication, logging, validation, or modifying the response before sending it to the client.
Question: What is the purpose of the "os" module in Node.js?
Answer:The "os" module in Node.js provides methods for interacting with the operating system on which the Node.js application is running. It allows you to retrieve information about the system's hardware, network interfaces, operating system version, and provides utilities for handling paths, resolving hostnames, managing processes, and more.
Question: How can you handle errors in Node.js applications?
Answer:Errors in Node.js applications can be handled using techniques such as error handling middleware, using try-catch blocks, using error events on streams or sockets, logging errors to a centralized error tracking system, or using error-handling libraries like "express-error-handler" or "errorhandler". Proper error handling is essential for handling unexpected issues, preventing crashes, and providing meaningful error messages to users for debugging and troubleshooting.
Question: What are the best practices for writing secure Node.js applications?
Answer:Some best practices for writing secure Node.js applications include:
- Validating and sanitizing all user input to prevent injection attacks and other security vulnerabilities.
- Implementing proper authentication and authorization mechanisms to protect against unauthorized access.
- Using secure password storage techniques, such as bcrypt or scrypt, to protect user passwords.
- Keeping all dependencies and libraries up-to-date to prevent known vulnerabilities.
- Implementing input validation and output encoding to prevent cross-site scripting (XSS) and cross-site request forgery (CSRF) attacks.
- Using HTTPS and SSL/TLS for secure communication over the network.
- Implementing security headers in HTTP responses, such as Content Security Policy (CSP), to prevent code injection attacks.
- Implementing rate limiting and throttling to protect against brute force attacks and DDoS attacks.
- Restricting access to sensitive files and resources on the server.
- Regularly auditing and monitoring the application for security vulnerabilities and fixing them promptly.