How to get started in React Native with typescript
Getting started with React Native and TypeScript is a great way to build powerful and performant mobile apps. This guide provides an overview of what you need to get started, including installing the React Native CLI, configuring development environment, and creating a new app with TypeScript. After completing this tutorial, you'll have the knowledge to start building React Native apps with TypeScript.
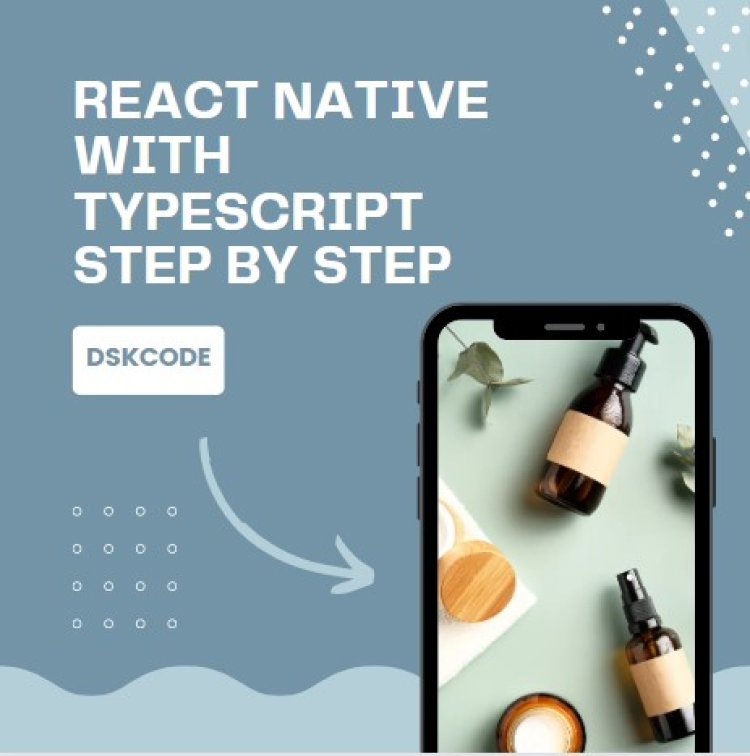
I can help you set up a React Native project with TypeScript step-by-step. Here's a detailed explanation:
Step 1: Install Node.js and React Native
First, you need to have Node.js installed on your machine. You can download and install it from the official Node.js website (https://nodejs.org/).
Next, you need to install React Native globally on your machine. Open a terminal or command prompt and run the following command:
Step 2: Create a new React Native Project
Once you have React Native installed, you can create a new project using the react-native init command. Open a terminal or command prompt and run the following command:
Replace "MyReactNativeApp" with the name you want to give to your project.
Step 3: Install TypeScript
Next, you need to install TypeScript as a development dependency in your project. Navigate to your project directory in the terminal or command prompt, and run the following command:
This will install TypeScript, as well as the TypeScript declaration files for React and React Native.
Step 4: Set up TypeScript Configuration
After installing TypeScript, you need to set up a TypeScript configuration file in your project. Create a new file named tsconfig.json in the root of your project folder, and add the following content:
"compilerOptions": {
"target": "esnext",
"moduleResolution": "node",
"allowSyntheticDefaultImports": true,
"jsx": "react-native",
"noEmit": true,
"strict": true,
"esModuleInterop": true,
"module": "esnext",
"baseUrl": "./",
"paths": {
"*": ["node_modules/*"],
"@/*": ["src/*"]
}
},
"exclude": [
"node_modules",
"babel.config.js",
"metro.config.js",
"jest.config.js",
"e2e",
"android",
"ios"
]
}
This configuration specifies the TypeScript compiler options for your project, including the target version, module resolution, JSX syntax, and more.
Step 5: Update File Extensions
In a TypeScript project, you need to rename your JavaScript files to .ts or .tsx extension. For example, you can rename App.js to App.tsx in your project's root folder.
Step 6: Update App.tsx
Open the App.tsx file in your project's root folder, and update it to use TypeScript syntax. For example, you can update it like this:
import { View, Text } from 'react-native';
const App: React.FC = () => {
return (
<View>
<Text>Hello, World!</Text>
</View>
);
};
export default App;
Note that we have specified the type of the App component as React.FC, which stands for "Function Component".
Step 7: Start the React Native Metro Bundler
You can start the Metro Bundler, which is the JavaScript bundler used in React Native, by running the following command in the terminal or command prompt:
Step 8: Build and Run the Project
You can now build and run your React Native project with TypeScript. Open a new terminal or command prompt, navigate to your project directory, and run the following command:
For iOS:
For Adroid:
This will compile and run your React Native app with TypeScript in the respective emulator or device.
Congratulations! You have successfully set up a React Native project with TypeScript. You can now start writing your React Native components with TypeScript's static typing, which provides better code completion, error checking, and overall development experience.
Note: If you encounter any issues during the setup process, make sure to check the official documentation for React Native and TypeScript for the latest updates and troubleshooting tips. Happy coding!
simple React Native app written in TypeScript:
import React from 'react';
import { View, Text, StyleSheet, TouchableOpacity } from 'react-native';
// Define Props interface for the App component
interface Props {}
const App: React.FC
const handlePress = () => {
console.log('Button pressed');
};
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, World!</Text>
<TouchableOpacity style={styles.button} onPress={handlePress}>
<Text style={styles.buttonText}>Press me</Text>
</TouchableOpacity>
</View>
);
};
// Define styles using StyleSheet.create
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 24,
marginBottom: 16,
},
button: {
backgroundColor: 'blue',
padding: 12,
borderRadius: 8,
},
buttonText: {
color: 'white',
fontSize: 18,
},
});
export default App;
In this example, we define an App component using TypeScript, which is a functional component with the React.FC type. We also define the Props interface for the App component, although it is currently empty in this example.
The App component renders a simple view with a text and a button using the View, Text, and TouchableOpacity components from React Native. We also define styles for the components using the StyleSheet.create method to ensure type-checking and autocompletion for the styles.
The handlePress function is a callback function that gets called when the button is pressed, and it logs a message to the console.
Note that in TypeScript, you can also define prop types using interfaces or type aliases to specify the expected props for your components, and TypeScript will provide type checking and autocompletion for props as well. Additionally, TypeScript helps catch potential type-related bugs and provides better code completion and error checking during development, making it a powerful tool for building robust and reliable React Native apps.