Python Snake Game Coding from Scratch
This Python Snake Game is a fun and interactive way to learn the basics of coding with Python. Players will use their knowledge of Python to control a virtual snake to collect food and avoid obstacles. This game is perfect for both experienced coders and those new to Python programming.
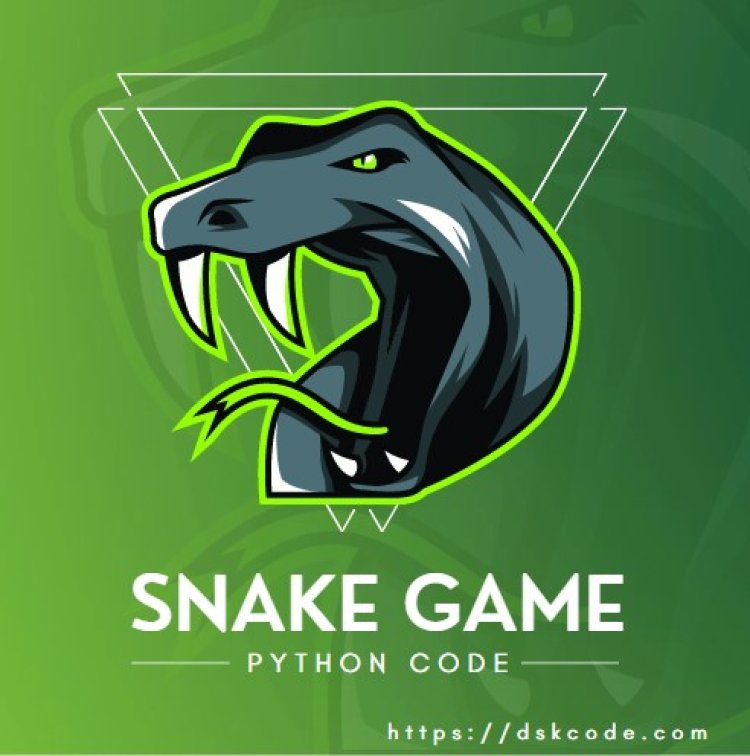
Importing Libraries: The code starts by importing the necessary libraries, including pygame, sys, and random. Pygame is a popular library used for creating 2D games in Python, while sys is used for system-level operations, and random is used for generating random numbers.
Initializing Pygame: The pygame.init() function is called to initialize the pygame library and set up the game window.
Setting up Game Window: The window_width and window_height variables define the size of the game window. The pygame.display.set_mode() function is called to create the game window with the specified width and height. The pygame.display.set_caption() function is used to set the caption for the game window.
Setting up Game Variables: The code sets up various game variables, such as snake_block_size, snake_speed, snake_color, snake_list, snake_length, food_color, food_block_size, food_x, food_y, and direction. These variables store important information about the game, such as the size and speed of the snake, the color of the snake and food, the position of the food, and the direction of the snake's movement.
Defining Functions: Two functions are defined - draw_snake() and draw_food(). The draw_snake() function takes the snake_list as input and draws the snake on the game window by iterating through the segments of the snake and drawing rectangles for each segment using the pygame.draw.rect() function. The draw_food() function takes the food_x and food_y as input and draws the food on the game window using the pygame.draw.rect() function.
Game Loop: The code enters into an infinite game loop, which constantly updates the game window and handles the game events.
Handling Events: The pygame.event.get() function is called to get the list of events that occurred in the game, such as keyboard inputs or mouse clicks. The for loop iterates through the list of events and checks for the pygame.QUIT event, which indicates that the game window was closed. If this event is detected, the pygame.quit() and sys.exit() functions are called to close the game window and exit the game.
Handling Snake Movement: The pygame.key.get_pressed() function is called to get the list of keys that are currently being pressed. The for loop iterates through the list of keys and updates the direction variable based on the arrow keys pressed by the player. The direction variable determines the movement direction of the snake.
Updating Snake's Position: The code updates the position of the snake's head based on the current direction. The head of the snake is updated by creating a new segment at the front of the snake_list, which stores the positions of all the segments of the snake. The previous segments are shifted one position forward in the list.
Checking for Collision with Food: The code checks if the snake's head has collided with the food by comparing its position with the position of the food. If a collision is detected, a new position for the food is generated randomly within the game window, and the snake's length is increased by 1.
Updating Snake's Length: If the length of the snake's list is greater than the desired snake_length, the last segment (tail) of the snake is removed from the list using the del statement. This ensures that the snake maintains its desired length.
Checking for Collision with Walls or Itself: The code checks if the snake's head has collided with the walls of the game window or with its own body. If a collision is detected, the pygame.quit() and sys.exit() functions are called to close the game window and exit the game.
Updating Game Window: Thecode updates the game window by filling it with the background color using the pygame.display.fill() function. Then, the draw_snake() function is called to draw the snake on the game window based on the updated position of its segments. The draw_food() function is also called to draw the food on the game window based on its current position.
Controlling Game Speed: The pygame.time.Clock() function is called to create a clock object that helps control the game speed. The clock.tick(snake_speed) function is called to limit the frame rate of the game, ensuring that the game runs at the desired speed.
Displaying Score: The code displays the score on the game window using the pygame.font.SysFont() function to create a font object, and then calling the render() function to render the score text. The score is updated based on the length of the snake's list minus the initial length.
Game Over: If the game is over due to collision with walls or the snake's body, the code displays a game over message on the game window using the pygame.font.SysFont() function to create a font object, and then calling the render() function to render the game over text. The game loop breaks, and the pygame.quit() and sys.exit() functions are called to close the game window and exit the game.
Running the Game: Finally, the pygame.display.update() function is called to update the game window with all the changes made in the current frame, and the game loop continues to run until the game is over or the window is closed.
Step 1: Import necessary libraries
import sys
import random
In this step, we import the required libraries: pygame for creating the game window and handling game events, sys for system-level operations, and random for generating random positions for the food.
Step 2: Initialize Pygame
This step initializes Pygame and prepares it for use in the game.
Step 3: Set up game window
window_height = 400
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption('Snake Game')
Here, we define the dimensions of the game window and create a window using pygame.display.set_mode(). We also set a title for the window using pygame.display.set_caption().
Step 4: Set up game variables
snake_speed = 10
snake_color = (0, 255, 0)
snake_list = []
snake_length = 1
food_color = (255, 0, 0)
food_block_size = 20
food_x = round(random.randrange(0, window_width - food_block
_height - food_block_size) / 20) * 20
In this step, we define various game variables, such as the size and color of the snake, the size and color of the food, the initial position of the food (randomly generated), and the initial state of the snake.
Step 5: Define functions for drawing snake and food
for x, y in snake_list:
pygame.draw.rect(window, snake_color, (x, y, snake_block_size, snake_block_size))
def draw_food(food_x, food_y):
pygame.draw.rect(window, food_color, (food_x, food_y, food_block_size, food_block_size))
Here, we define two functions for drawing the snake and the food on the game window. The draw_snake() function takes in the list of snake segments as input and draws each segment on the window using the pygame.draw.rect() function. The draw_food() function takes in the current position of the food and draws it on the window.
Step 6: Run game loop
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Handle snake movement
keys = pygame.key.get_pressed()
for key in keys:
if keys[pygame.K_LEFT]:
# update snake's x-coordinate
if keys[pygame.K_RIGHT]:
# update snake's x-coordinate
if keys[pygame.K_UP]:
# update snake's y-coordinate
if keys[pygame.K_DOWN]:
# update snake's y-coordinate
# Update game window
window.fill((0, 0, 0))
draw_snake(snake_list)
draw_food(food_x, food_y)
pygame.display.update()
# Check for collision with food
# Update snake's length and position
# Check for collision with walls or itself
This is the main game loop where the game logic will be implemented. It continuously runs and updates the game window, handles user input for snake movement, checks for collision with food, updates the snake's length and position, and checks for collision with walls or itself. You will need to complete the snake movement logic, collision detection, and other game mechanics based on your desired game rules.
This code creates a simple snake game where the player controls a snake
import pygame
import sys
import random
pygame.init()
# Set up game window
window_width = 400
window_height = 400
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption('Snake Game')
# Set up game variables
snake_block_size = 20
snake_speed = 10
snake_color = (0, 255, 0)
snake_list = []
snake_length = 1
food_color = (255, 0, 0)
food_block_size = 20
food_x = round(random.randrange(0, window_width - food_block_size) / 20) * 20
food_y = round(random.randrange(0, window_height - food_block_size) / 20) * 20
direction = "RIGHT"
# Define functions for drawing snake and food
def draw_snake(snake_list):
for x, y in snake_list:
pygame.draw.rect(window, snake_color, (x, y, snake_block_size, snake_block_size))
def draw_food(food_x, food_y):
pygame.draw.rect(window, food_color, (food_x, food_y, food_block_size, food_block_size))
# Run game loop
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Handle snake movement
keys = pygame.key.get_pressed()
for key in keys:
if keys[pygame.K_LEFT]:
direction = "LEFT"
if keys[pygame.K_RIGHT]:
direction = "RIGHT"
if keys[pygame.K_UP]:
direction = "UP"
if keys[pygame.K_DOWN]:
direction = "DOWN"
# Update snake's position
if direction == "LEFT":
head = [snake_list[0][0] - snake_block_size, snake_list[0][1]]
if direction == "RIGHT":
head = [snake_list[0][0] + snake_block_size, snake_list[0][1]]
if direction == "UP":
head = [snake_list[0][0], snake_list[0][1] - snake_block_size]
if direction == "DOWN":
head = [snake_list[0][0], snake_list[0][1] + snake_block_size]
snake_list.insert(0, list(head))
# Check for collision with food
if snake_list[0][0] == food_x and snake_list[0][1] == food_y:
food_x = round(random.randrange(0, window_width - food_block_size) / 20) * 20
food_y = round(random.randrange(0, window_height - food_block_size) / 20) * 20
snake_length += 1
# Update snake's length
if len(snake_list) > snake_length:
del snake_list[-1]
# Check for collision with walls or itself
for segment in snake_list[1:]:
if segment == head:
pygame.quit()
sys.exit()
if head[0] < 0 or head[0] >= window_width or head[1] < 0 or head[1] >= window_height:
pygame.quit()
sys.exit()
# Update game window
window.fill((0, 0, 0))
draw_snake(snake_list)
draw_food(food_x, food_y)
pygame.display.update()
pygame.time.Clock().tick(snake_speed)