Using the React useDebugValue Hook
Learn how to use the React useDebugValue hook to add custom information to the React DevTools Profiler. Gain an understanding of the useDebugValue hook and how to use it to debug React components.
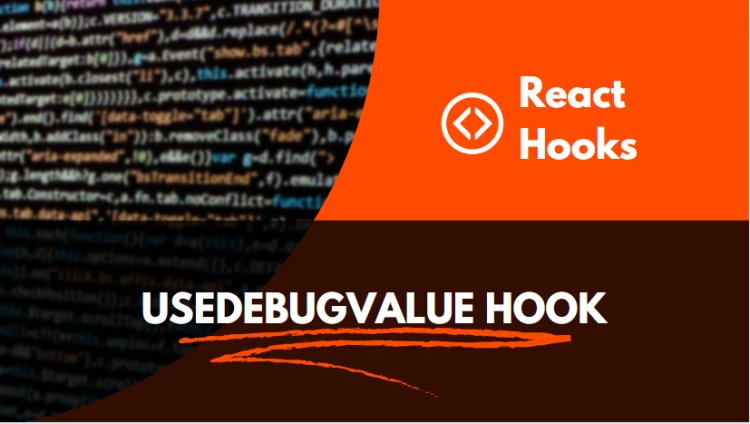
Table of Content:
I. Introduction
A. What is the React useDebugValue Hook?
B. What are the Benefits of Using the React useDebugValue Hook?
II. How to Use the React useDebugValue Hook
A. Setting Up the Hook
B. Implementing the Hook
C. Using the Hook
III. Tips for Using the React useDebugValue Hook
A. Naming the Hook
B. Utilizing the Hook in Debugging
C. Using the Hook in Production
IV. Conclusion
A. Summary of the React useDebugValue Hook
B. Benefits of Using the Hook
Introduction:
The useDebugValue hook is a React feature which allows you to display a custom value in the React DevTools for a given component instance. This is especially useful when debugging complex or dynamic components. With useDebugValue you can display a meaningful label for each instance of a component, which can make it easier to identify the source of the issue.
The useDebugValue hook is part of the React DevTools API. It allows you to specify a custom label for a given component instance, that will be displayed in the React DevTools. This can be especially useful when debugging complex or dynamic components, as you can easily distinguish between different instances.
To use the useDebugValue hook, you can simply pass a label string as the first argument when calling the hook. The label string will then be displayed in the React DevTools in the “Custom” column. This can help you quickly identify the source of any issues, or simply provide more context when looking at the DevTools.
The useDebugValue hook can also be used to display more detailed information in the “Custom” column. You can pass an object as the second argument when calling the hook, which can contain any additional data that you want to display. This can be especially useful when debugging complex components, as you can quickly view all the relevant data in the DevTools.
Overall, the useDebugValue hook is a very useful feature for debugging React components. It can help you quickly identify the source of an issue, or provide more context when looking at the React DevTools.
How to Use the React useDebugValue Hook
1. Import the useDebugValue hook from React:
import { useDebugValue } from 'react';
2. Declare the useDebugValue hook and pass it a value to be used for debugging. This value can be a string, number, or object.
const [value, setValue] = useDebugValue('Debugging Value');
3. The value of the hook will be displayed in the React DevTools when the component is selected in the tree.
4. Optionally, you can provide a function as a second argument to return a formatted value for the DevTools. This function will be called whenever the value of the hook changes.
const [value, setValue] = useDebugValue('Debugging Value', value => {
return `Debugging Value is currently set to ${value}`;
});
import { useDebugValue } from 'react';
const MyComponent = () => {
const someValue = useSomeValue();
useDebugValue(someValue);
return <div>{someValue}</div>;
};
Tips for Using the React useDebugValue Hook
If you're using the React useDebugValue hook to display a value in the React DevTools, there are a few tips to keep in mind.
First, useDebugValue only accepts strings or numbers as values. If you try to pass in an object, it will be automatically stringified by React DevTools.
Second, the value you pass into useDebugValue will be displayed in the React DevTools as a tooltip. If you want to display a more complex value, you can use the useDebugValueFormatters hook.
Finally, keep in mind that useDebugValue will only be called when the component is rendered in development mode. In production mode, the value will not be displayed in the React DevTools.
Conclusion
The useDebugValue hook is a great tool for helping developers track and debug the values within a React application. By providing a custom label and a custom value, developers can quickly identify the values of components and make sure that they are functioning as intended. Additionally, the useDebugValue hook can be used to track the performance of components, allowing developers to optimize the application and make sure it is running as efficiently as possible.
Example:
import React, { useState, useDebugValue } from 'react';
const App = () => {
const [value, setValue] = useState(0);
// useDebugValue hook allows us to display custom values
// inside React DevTools
useDebugValue(`Value is ${value}`);
return (
<div>
<p>Value: {value}</p>
<button onClick={() => setValue(value + 1)}>Increment</button>
</div>
);
};
export default App;