how to convert seconds to hour minute second in react js
very simple and easy way to convert seconds into HH-MM-SS formatted time. Here in this example code, I have specified the seconds as 5000 and it returns the 1 as the hour, 23 as minutes and 15 as seconds. It returns the output 01:23:20.
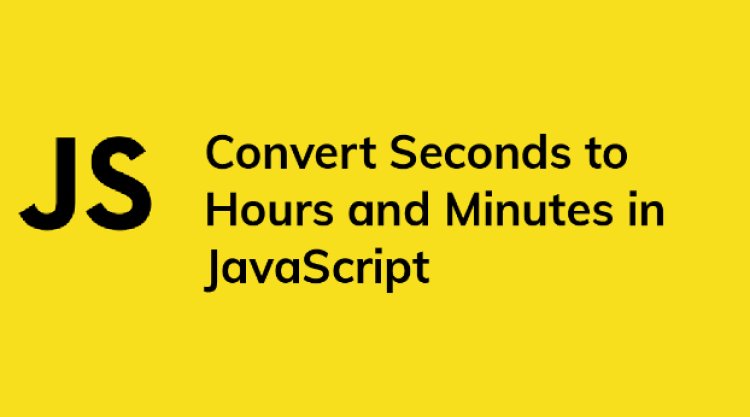
Discuss how to convert seconds to hour minute second in react js.
Formula
- time in seconds = time in minutes * 60 = time in hours × 3600.
- time in minutes = time in seconds / 60 = time in hours × 60.
- time in hours = time in minutes / 60 = time in seconds / 3600.
To convert seconds to hours, minutes, and seconds, divide the number of seconds by 3600.
For example, if there are 30 seconds in a minute, then the conversion would be 1/3600 or 0.0036.
Demonstrate how to use Reactjs to convert seconds to hour minute second.
function secondsToHms(d) {
d = Number(d);
var h = Math.floor(d / 3600);
var m = Math.floor(d % 3600 / 60);
var s = Math.floor(d % 3600 % 60);
var hDisplay = h > 0 ? h + (h == 1 ? " hour, " : " hours, ") : "";
var mDisplay = m > 0 ? m + (m == 1 ? " minute, " : " minutes, ") : "";
var sDisplay = s > 0 ? s + (s == 1 ? " second" : " seconds") : "";
return hDisplay + mDisplay + sDisplay;
}