Using the React useMemo Hook to Optimize Performance
Learn how to use the React useMemo hook to optimize performance in your applications. Find out how to effectively store and access data in memory, and leverage the useMemo hook to efficiently manage and update your components.
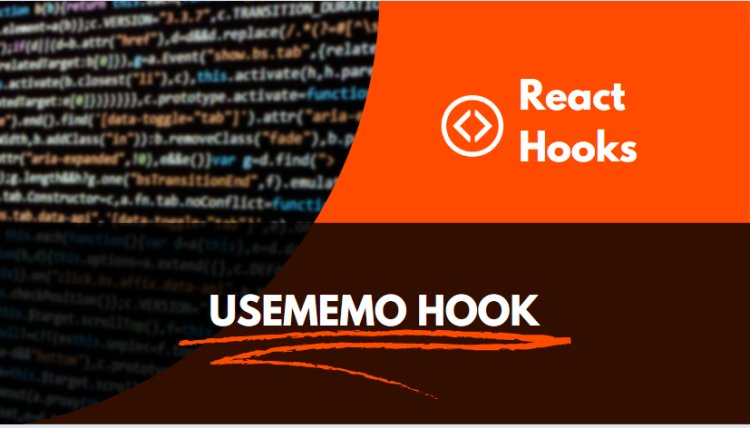
What is the React useMemo hook?
React's useMemo hook is a built-in Hook that allows you to optimize the rendering performance of your React components. It helps to ensure that expensive calculations or re-rendering of expensive components are only performed when the values used in the calculations have actually changed.
The useMemo hook takes in two arguments, the first being a function that returns a value, and the second being an array of values which are used as dependencies for the first argument. The useMemo hook will remember the result of the function, and then only execute the function again if one of the dependencies has changed. This allows for optimization of expensive calculations and re-rendering of expensive components.
For example, if you have a React component that needs to frequently recalculate a value based on some input from the user, you can use the useMemo hook to make sure that the calculation only happens when the input has actually changed. This can help prevent unnecessary re-rendering of the component which can help improve the performance of your React application.
import React, { useMemo } from 'react';
const Example = () => {
// Declare a new state variable, which we'll call "count"
const [count, setCount] = useState(0);
// useMemo will only recompute the memoized value when one of the
// dependencies has changed.
const expensive = useMemo(() => {
console.log('Recomputing');
let result = 0;
for (let i = 0; i < count; i++) {
result += i;
}
return result;
}, [count]); // Only re-compute the result when count changes
return (
<div>
<h1>{expensive}</h1>
<button onClick={() => setCount(count + 1)}>
Increment
</button>
</div>
);
};
What are the benefits of using the useMemo hook?
1. Improved performance: useMemo helps to optimize performance by memoizing a function or a value and caching its results. This avoids unnecessary re-computations or re-evaluations when the values used for the computation have not changed.
2. Improved readability: useMemo helps make code more readable as it allows developers to separate logic from components and avoid deeply nested component trees.
3. Improved scalability: useMemo helps to scale code by allowing developers to easily reuse logic and values across components. This helps to reduce code duplication and maintain modularity.
How can you use the useMemo hook to optimize performance?
The useMemo hook is a React hook that allows you to memoize a function's return value. This means that if the same inputs are passed to the function, the previously computed value is returned instead of re-computing the value. This can significantly improve the performance of a React application. For example, you could use useMemo to cache expensive calculations, such as data fetching, deep object comparisons, and heavy DOM calculations. By memoizing these calculations, you can ensure that the same calculations are not unnecessarily repeated, helping to improve the performance of your application.
What are some things to keep in mind when using the useMemo hook?
1. Use the useMemo hook sparingly as it can have a negative impact on performance.
2. Make sure that the function passed to useMemo is a pure function that does not cause any side effects.
3. Make sure to pass the same dependencies to useMemo each time you want it to be invoked.
4. If the data you are memoizing is expensive to compute, consider using useMemo to store the result.
5. If the data you are memoizing is small and will not change frequently, consider using a regular variable instead.
6. Make sure to use the latest version of React when using useMemo.
How can you use the useMemo hook to improve the performance of your React applications?
The useMemo hook can be used to improve the performance of React applications by caching values that are expensive to compute. This means that instead of recomputing the same value every time a component is rendered, the value is only calculated once and stored in the cache. This saves time and resources, as well as improving the overall performance of the application.
usememo react hook example program
import React, { useState, useMemo } from 'react';
const App = () => {
const [number, setNumber] = useState(0);
const [text, setText] = useState('');
const expensiveOperation = () => {
console.log('Running expensive operation!');
let sum = 0;
for (let i = 0; i < number; i++) {
sum += i;
}
return sum;
};
const memoizedValue = useMemo(() => expensiveOperation(), [number]);
return (
<div>
<h1>React useMemo Hook Example</h1>
<input
type="number"
value={number}
onChange={(e) => setNumber(e.target.value)}
/>
<input
type="text"
value={text}
onChange={(e) => setText(e.target.value)}
/>
<br />
<br />
<p>Number: {number}</p>
<p>Text: {text}</p>
<p>Memoized value: {memoizedValue}</p>
</div>
);
};
export default App;