Understanding the useLayoutEffect React Hook
Discover how to use the useLayoutEffect React hook to efficiently manage component layout and state in your React applications. Learn how to use useLayoutEffect to create smooth, performant UIs that are responsive to user interactions.
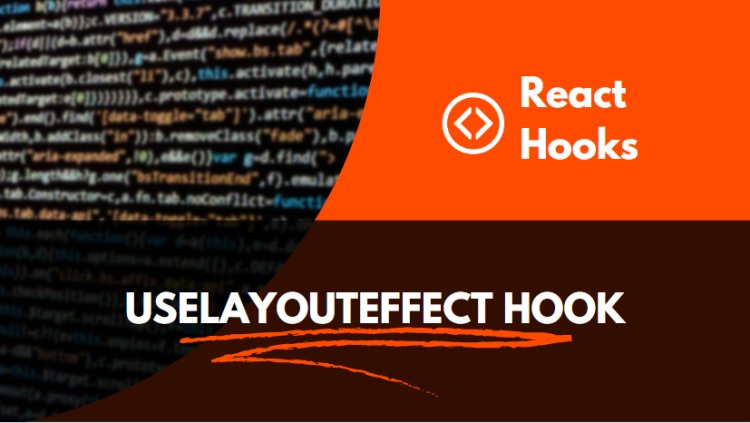
What is the useLayoutEffect React Hook?
The useLayoutEffect React Hook is used to trigger a function after a component has been laid out in the DOM (Document Object Model). This hook is used to synchronize the DOM with other state changes, such as the ones that come from a Redux or Context store.
It is important to note that useLayoutEffect should not be used in place of React's useEffect hook. Unlike useEffect, useLayoutEffect runs synchronously and will block other updates until it has completed. This can lead to performance issues, so useLayoutEffect should be used sparingly and only when absolutely necessary.
The useLayoutEffect hook is ideal for situations where you need to ensure that the DOM is updated with the most up-to-date state before it is read or rendered. This can be useful for updating the position of an element based on a Redux store or for responding to changes in the DOM.
In general, the useLayoutEffect hook should be used sparingly and only when absolutely necessary. It is important to understand the implications of using this hook and to ensure that its use will not negatively impact the performance of your application.
import { useLayoutEffect } from 'react';
const MyComponent = () => {
useLayoutEffect(() => {
// Do something
});
return <div>MyComponent</div>;
}
How can the useLayoutEffect React Hook be used to improve performance?
The useLayoutEffect React Hook can be used to improve performance by batching DOM mutations together and applying them before the browser has a chance to paint. This can be particularly useful for doing things like applying CSS styles or reading layout information, which can be expensive operations.
Layout effects run after the browser has painted, so they can't be used to prevent flicker. However, they can be used to clean up after an effect, or to perform DOM mutations that can't be batched (such as reading layout information).
One limitation of using useLayoutEffect is that it doesn't have a cleanup function like useEffect does. This means that if you need to perform a side effect that doesn't have a corresponding cleanup effect, you'll need to use a ref to store the side effect's return value and call the cleanup function manually.
What are some potential drawbacks of using the useLayoutEffect React Hook?
The useLayoutEffect React Hook is a powerful tool for optimizing the performance of React applications and for providing a finer control over the timing of certain operations. However, it also has some potential drawbacks that must be taken into account when using it.
First, useLayoutEffect has a tendency to cause re-rendering of components more often. This can lead to increased CPU usage and other performance issues. Additionally, because it runs synchronously after the React render cycle, it can cause unexpected behavior if not used correctly.
Second, useLayoutEffect can lead to increased complexity in the code, as it has to be used carefully to ensure that the order of the operations executed is correct. This can make the code harder to read and maintain, especially for novice developers.
Finally, useLayoutEffect can also cause issues with server-side rendering, as the operations might not be completed when the page is rendered. This can lead to unexpected results that can be hard to track down and fix.
Overall, useLayoutEffect can be a very useful tool for improving the performance of React applications, but it must be used cautiously to avoid potential pitfalls.
How can the useLayoutEffect React Hook be used to avoid potential errors?
If you're using React, you're probably familiar with the concept of 'lifecycle hooks.' These are special functions that allow you to control when certain things happen in your component.
One of the most commonly used lifecycle hooks is the useLayoutEffect React hook. This hook is invoked immediately after a component is mounted or updated. It's often used to perform side effects, such as setting up a subscription or fetching data.
However, useLayoutEffect comes with a potential pitfall: it's easy to forget to clean up after yourself. If you forget to unsubscribe from a subscription or cancel a fetch, you may end up with a memory leak.
To avoid this, it's important to always remember to cleanup after yourself when using useLayoutEffect. Make sure to unsubscribe from any subscriptions and cancel any fetches before the component unmounts. If you need to perform a side effect that doesn't require cleanup, you can use the useEffect React hook instead.
What are some best practices for using the useLayoutEffect React Hook?
1. Use layout effects sparingly: Layout effects should only be used when necessary, as they can be computationally expensive to run. If a task can be accomplished with a regular effect, it should be used instead.
2. Use useMemo to memoize expensive calculations: If a layout effect contains an expensive calculation, it should be memoized using the useMemo hook. This will ensure that the calculations are only run when necessary and not on every render.
3. Avoid React mutations: Mutating the DOM directly with React can lead to unexpected bugs, so it should be avoided whenever possible.
4. Don’t cause infinite loops: Layout effects can cause infinite loops if not used properly, so it’s important to be aware of this when writing them.
5. Ensure that any side effects happen after the layout is complete: If there are any side effects that need to happen after the layout is completed, they should be wrapped in a useLayoutEffect callback to ensure they are executed only after the layout is complete.
6. Don’t rely on the order of layout effects: Layout effects don’t always run in the order they are declared, so it’s important to make sure that each one is independent and that it doesn’t rely on the results of another.
Example:
import React, { useLayoutEffect } from 'react';
const Example = () => {
const [width, setWidth] = React.useState(0);
useLayoutEffect(() => {
const updateWidth = () => setWidth(window.innerWidth);
window.addEventListener('resize', updateWidth);
updateWidth();
return () => window.removeEventListener('resize', updateWidth);
}, []);
return (
<div>
<p>Window width is {width}</p>
</div>
);
};
export default Example;