Using the useCallback React Hook to Optimize Performance
Learn how to use the useCallback React Hook to improve the performance of your React applications by avoiding unnecessary re-renders. Discover the benefits and limitations of using useCallback, and see how to implement it in your code.
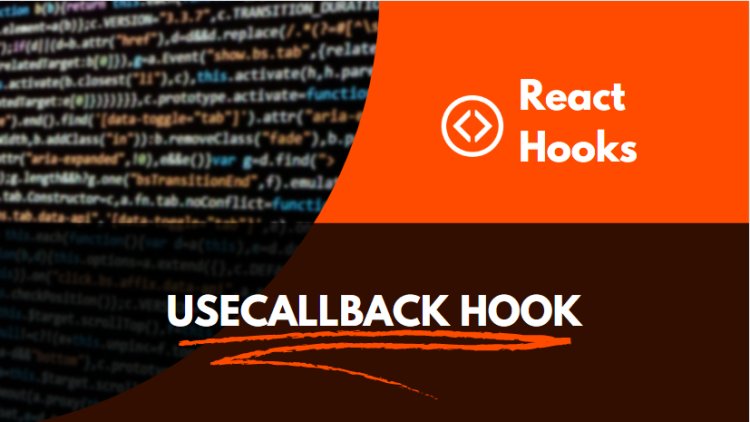
What is the useCallback React Hook and why is it important?
The useCallback React Hook is a function that allows you to memoize (cache) the result of a function that changes over time. This is important because it helps to optimize the performance of React applications by preventing unnecessary re-renders. This is especially useful for optimizing expensive calculations or API calls that are triggered by user interaction. By memoizing the result of the function, React will only re-run the function when one of its dependencies has changed, rather than running it every time the component re-renders.
How to use the useCallback React Hook to optimize performance.
If you’re familiar with React, you’ve probably used the useCallback React Hook before. If not, useCallback is a React Hook that allows you to memoize the return value of a function. In other words, it allows you to cache the result of a function so that you don’t have to recalculate it every time it is invoked.
This can be especially useful when you have a performance-intensive function, such as a function that renders a large list or performs a deep comparison. By memoizing the result of the function, you can ensure that it is only ever invoked once, which can dramatically improve the performance of your React application.
So how do you use the useCallback React Hook? First, you need to define a function that you want to memoize. For example, let’s say you have a function that renders a list of items:
const renderListItems = (items) => {
return items.map((item, index) => (
{item}));
}
Next, you can use the useCallback React Hook to memoize the function:
const memoizedRenderListItems = useCallback(renderListItems, []);
The first argument is the function that you want to memoize, and the second argument is an array of dependencies. In this case, we’re passing an empty array because the renderListItems function doesn’t have any dependencies.
Now, every time you call the memoizedRenderListItems function, it will return the cached result of the renderListItems function. This means that the renderListItems function will only be invoked once, even if it is called multiple times.
You can also use the useCallback React Hook to memoize a function with dependencies. For example, let’s say you have a function that compares two items:
const compareItems = (item1, item2) => {
return item1 === item2;
}
You can use the useCallback React Hook to memoize this function as well:
const memoizedCompareItems = useCallback((item1, item2) => compareItems(item1, item2), [compareItems]);
This time, we’re passing the compareItems function as a dependency because the memoizedCompareItems function depends on it. This means that the compareItems function will only be invoked once, even if the memoizedCompareItems function is called multiple times.
So there you have it! The useCallback React Hook is a great way to improve the performance of your React application by memoizing the result of a function.
What are some common mistakes when using the useCallback React Hook?
1. Not passing the dependencies correctly - The useCallback hook will only return a memoized version of the callback if its dependencies have not changed. If dependencies are not passed correctly, the hook will return a new callback every time.
2. Not using useCallback when appropriate - Using useCallback is not always necessary, and can have a negative impact on performance if it is used unnecessarily.
3. Overusing useCallback - While useCallback can be a great tool to improve performance, it can become a hindrance if it is overused. If the same callback is being used in multiple places, it may be better to move the logic outside of the useCallback hook.
How to avoid those mistakes and optimize performance even further
There are a few basic principles that can help you avoid making common mistakes when optimising your code for performance.
Firstly, try to avoid unnecessary work. If a piece of code doesn't need to be run, don't run it. This might seem obvious, but it's easy to forget when you're optimising code.
Secondly, make sure you're using the most efficient algorithms and data structures for the task at hand. Don't use a complex algorithm when a simpler one will do, and don't use a inefficient data structure when a more efficient one is available.
Finally, don't be afraid to profile your code to identify bottlenecks. Once you've found a bottleneck, focus your optimisation efforts on that area of the code.
Follow these basic principles and you'll be well on your way to writing high-performance code.
Examples of how to use the useCallback React Hook in different situations.
The useCallback React Hook is a useful way to optimize your React application. It allows you to memoize values so that they are only recalculated when certain dependencies change. This can be a performance boost in many situations.
One example of where this might be useful is if you have a component that renders a list of items. Each item in the list has a unique id. The component also has a button that adds a new item to the list. When the button is clicked, the component needs to calculate the new id for the new item. However, this calculation only needs to happen if the list of items has changed. If the list of items is the same, then the id can be reused.
Another example where this might be useful is when you have a form with a lot of input fields. Each time the user types something in a field, the entire form needs to be re-rendered. However, if you use the useCallback React Hook, you can memoize the form values. This means that the form will only be re-rendered if the values have actually changed.
There are many other potential uses for the useCallback React Hook. These are just a few examples. Experiment and see if you can find other ways to use it in your own applications.
1. Caching Callback Functions:
const memoizedCallback = useCallback(() => {
doSomething(a, b);
}, [a, b]);
2. Passing Callback Functions as Props:
const ParentComponent = () => {
const memoizedCallback = useCallback(() => {
doSomething(a, b);
}, [a, b]);
return <ChildComponent callback={memoizedCallback} />;
};
3. Setting Up Event Listeners:
const MyComponent = () => {
const handleClick = useCallback(() => {
console.log('Clicked!');
}, []);
useEffect(() => {
document.addEventListener('click', handleClick);
return () => {
document.removeEventListener('click', handleClick);
};
}, [handleClick]);
return <div>My Component</div>;
};
Example:
// example 1
import React, { useState, useCallback } from 'react';
const ExampleComponent = () => {
const [count, setCount] = useState(0);
// This will only be created once and won't be recreated when a re-render happens
const incrementCount = useCallback(() => {
setCount(count + 1);
}, [count]);
return (
<div>
<button onClick={incrementCount}>Click Me</button>
<p>{count}</p>
</div>
);
};
export default ExampleComponent;
// example 2
import React, { useState, useCallback } from 'react';
const ExampleComponent = () => {
const [inputValue, setInputValue] = useState('');
const [timesClicked, setTimesClicked] = useState(0);
// This will only be created once and won't be recreated when a re-render happens
const handleInputChange = useCallback(
(e) => {
setInputValue(e.target.value);
},
[inputValue]
);
// This will also only be created once and won't be recreated when a re-render happens
const handleButtonClick = useCallback(() => {
setTimesClicked(timesClicked + 1);
}, [timesClicked]);
return (
<div>
<input type="text" value={inputValue} onChange={handleInputChange} />
<button onClick={handleButtonClick}>Click Me</button>
<p>{timesClicked}</p>
</div>
);
};
export default ExampleComponent;