How to use Tailwind CSS in React Native With Example
Learn how to use Tailwind CSS in React Native with this easy-to-follow guide, complete with examples.
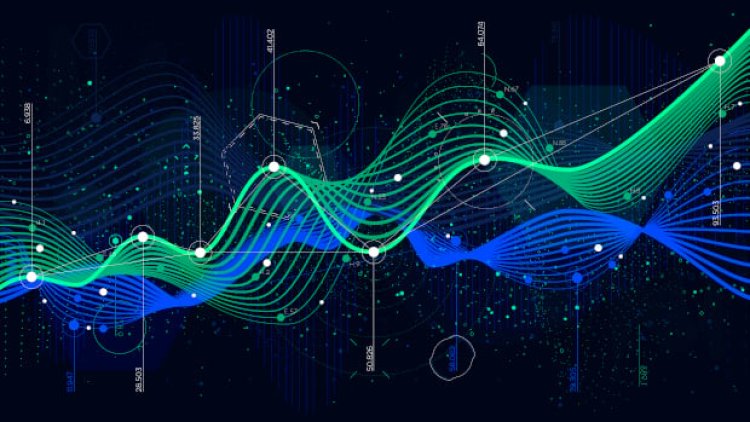
What is Tailwind CSS?
Tailwind CSS is a utility-first CSS framework for rapidly building custom designs.
A utility-first CSS framework is one that provides low-level utility classes that let you build completely custom designs without ever having to write a single line of CSS.
Tailwind is different from other CSS frameworks because it's not opinionated about how you should build your website. It just provides the building blocks you need to create whatever kind of design you want.
You can think of Tailwind as lego for CSS. It's like having a box of lego pieces that you can use to build anything you want.
Tailwind is also different from other CSS frameworks in that it doesn't include any pre-built components or design templates. This is intentional, as the focus is on providing low-level utility classes that you can use to build your own custom designs.
If you're looking for a CSS framework that will help you build custom designs quickly, without having to write a lot of CSS, Tailwind is a great option.
What are the benefits of using Tailwind CSS?
Tailwind CSS is a utility-first CSS framework that provides a highly composable set of low-level utility classes that make it easy to build complex, responsive user interfaces without having to write any CSS yourself.
The benefits of using Tailwind are:
1. You can build complex responsive interfaces without writing any CSS yourself.
2. Tailwind provides a large set of low-level utility classes that are easy to compose together, making it very flexible.
3. Tailwind is very well thought out and organized, making it easier to reason about and maintain your CSS.
4. Tailwind is very performant, as it uses atomic CSS classes which are very fast to render.
5. Tailwind has great documentation and a large community, making it easy to get started and find help when needed.
How to set up Tailwind CSS in React Native?
Assuming you have a React Native project set up, you can install Tailwind CSS via npm:
npm install tailwindcss
Once Tailwind CSS is installed, you need to create a configuration file. You can use the npx tailwind init command to create a basic configuration file, or you can create a file called tailwind.config.js in your project root with the following contents:
// tailwind.config.js module.exports = { purge: [], theme: { extend: {}, }, variants: {}, plugins: [], }
The purge option tells Tailwind CSS to remove unused styles from your CSS build. In most cases, you will want to use this option in production to keep your CSS build as small as possible.
The theme option allows you to customize the default Tailwind CSS theme. In this example, we are extending the default theme with some custom colors.
The variants option allows you to control which variants are generated for each CSS property. By default, Tailwind CSS generates all variants (e.g. responsive, hover, focus, etc).
The plugins option allows you to add additional Tailwind CSS plugins.
Once your configuration file is set up, you can create a CSS file in your src directory and import Tailwind CSS:
// src/tailwind.css @tailwind base; @tailwind components; @tailwind utilities;
You can then import this CSS file into your React Native project:
import './tailwind.css';
Now you can use Tailwind CSS classes in your React Native project!
How to use Tailwind CSS in React Native?
If you want to use Tailwind CSS in a React Native app, there are a few steps you need to take. First, you need to install the tailwindcss package from npm. Then, you need to create a tailwind.config.js file in your project's root directory. In this file, you will need to specify your Tailwind CSS configuration. Finally, you will need to import the tailwind.css file into your React Native app.
Once you have installed the tailwindcss package and created your tailwind.config.js file, you can import the tailwind.css file into your React Native app like this:
import 'tailwindcss/tailwind.css';
Now, you can use all of the Tailwind CSS classes in your React Native app.
Another Method : Inline Style
import React from "react";
import { StyleSheet, View, Text } from "react-native";
import tw from "tailwind-react-native-classnames";
export default function RNTailwind() {
return (
<View
style={[
tw`flex flex-row items-center`,
{
position: "fixed",
},
]}
>
<View
style={[
tw`flex flex-row justify-between items-center`,
{
position: "absolute",
},
]}
>
<Text
numberOfLines={1}
style={[tw`text-lg font-extrabold text-white`, styles.text]}
>
Hello
);
}
const styles = StyleSheet.create({
text: {
width: 120,
color: "#424242",
margin: 25,
},
});
What are some common Tailwind CSS classes?
Some common Tailwind CSS classes are:
-tw-flex
-tw-inline-flex
-tw-grid
-tw-inline-grid
-tw-table
-tw-table-cell
-tw-text
-tw-leading
-tw-tracking
-tw-text-sm
-tw-text-lg
-tw-text-xl
-tw-font-semibold
-tw-font-medium
-tw-break-normal
-tw-break-words
-tw-break-all
-tw-select-none
-tw-border
-tw-border-width
-tw-border-style
-tw-border-color
-tw-rounded
-tw-rounded-t
-tw-rounded-b
-tw-rounded-l
-tw-rounded-r
-tw-rounded-tl
-tw-rounded-tr
-tw-rounded-bl
-tw-rounded-br
-tw-px
-tw-py
-tw-p
-tw-m
-tw-mt
-tw-mr
-tw-mb
-tw-ml
-tw-mx
-tw-my
-tw-opacity
How to customize Tailwind CSS in React Native?
If you're looking to customize Tailwind CSS in React Native, there are a few things you'll need to do. First, you'll need to install the tailwindcss package from npm. Next, you'll need to create a tailwind.config.js file in your project's root directory. In this file, you'll need to specify your customizations.
Once you've done this, you can import the tailwind.css file into your React Native project. From there, you can use the styleSheet API to style your components. For more information on how to use Tailwind CSS in React Native, check out the official documentation.
What are some tips for using Tailwind CSS in React Native?
If you're looking to use Tailwind CSS in React Native, here are some tips to help you get started:
1. Use the style prop instead of the className prop.
2. Use the classnames package to help with managing class names.
3. Use inline styles instead of CSS stylesheets.
4. Use the Tailwind CLI tool to help with generating Tailwind CSS styles.
5. Use the Tailwind UI library to help with creating React Native components.