How to Use the useEffect Hook in React
Learn how to use the useEffect hook in React to perform side effects after a component is rendered. Understand why useEffect is important and how to correctly use it in your application.
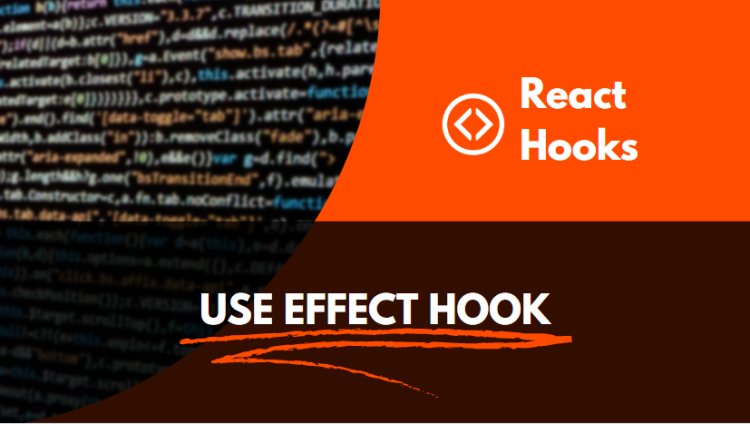
What is the useEffect hook?
The useEffect hook is a built-in React hook that allows developers to run side effects in functional components. It is a combination of the componentDidMount, componentDidUpdate, and componentWillUnmount lifecycle methods from class components. It is used to perform any side effects outside of the React component, such as data fetching, subscriptions, or manually changing the DOM.
The useEffect hook is a powerful hook that allows developers to perform side effects in a functional component. It is an alternative to componentDidMount, componentDidUpdate, and componentWillUnmount in a class component. It can be used for data fetching, setting up a subscription, and manually changing the DOM, among other things.
A common use of the useEffect hook is to fetch data from an API and update the component's state with the response.
Benefits of using this hook
The useEffect hook makes it easier for developers to perform side effects in functional components. This hook helps to keep the code clean and organized, and it also helps to improve performance since it can be used to run code only when certain conditions are met.
The useEffect hook is a powerful and versatile feature of React that lets developers run code at specific points in the component’s lifecycle. This hook provides a great way to manage side effects in a React application, such as data fetching, manually changing the DOM, or interacting with external APIs.
By using the useEffect hook, developers can ensure that their code is only run when certain conditions are met. For example, the hook can be used to trigger a data fetching operation when a component is mounted, or to update the DOM
How to use the hook
The useEffect hook is a built-in React hook that allows you to perform side effects in function components. It is a combination of componentDidMount, componentDidUpdate, and componentWillUnmount lifecycle methods in class components.
The useEffect hook takes in two arguments: a function and an array of dependencies. The function is the effect itself and is called when the component is mounted and re-rendered. The array of dependencies is an optional second argument that tells React when to call the effect. If the array is empty, the effect is called every time the component is re-rendered. If the array contains values, the effect is called only when the values in the array change.
When using the useEffect hook, it is important to note that the function passed as the first argument will be called after the render is committed to the screen. This means that the DOM will be updated before the effect is called. This is important to keep in mind when writing effects that interact with the DOM.
The useEffect hook is a powerful tool for managing side effects in React. It is important to remember that the effects should be used sparingly and only when they are necessary. Too many effects can slow down the performance of a React application
Example:
import React, { useState, useEffect } from 'react';
const App = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/todos')
.then((response) => response.json())
.then((data) => setData(data));
}, []);
return (
<div>
<ul>
{data.map((todo) => (
<li key={todo.id}>{todo.title}</li>
))}
</ul>
</div>
);
};
export default App;
Example program
React Hooks allow developers to write React components using functions and access state and lifecycle methods without writing a class. The useEffect hook is a powerful tool that makes it possible to access data from external sources such as APIs. This tutorial will show you how to use the useEffect hook in combination with the Fetch API to retrieve data from an external source. You will learn how to set up a fetch request, how to handle errors and how to use the data received from the server. Finally, you will see an example of how this can be used to create a dynamic list of items. By the end of this tutorial, you will have a better understanding of how to use the useEffect hook and the Fetch API to retrieve data in React Hooks.
import React, {useState, useEffect} from 'react';
function EmployeeSalary() {
const [employees, setEmployees] = useState([]);
const [salary, setSalary] = useState(0);
useEffect(() => {
fetch('/employees')
.then(res => res.json())
.then(data => {
setEmployees(data);
setSalary(calculateSalary(data));
})
.catch(err => console.log(err));
}, []);
const calculateSalary = (employees) => {
let salary = 0;
employees.forEach(employee => {
salary += employee.salary;
});
return salary;
}
return (
<div>
<h1>Employee Salary</h1>
<h2>Total Salary: {salary}</h2>
</div>
);
}
export default EmployeeSalary;