Exploring Powerful Features of react native vision camera: What You Need to Know
Learn how to integrate react native vision camera to create stunning visual effects in apps.
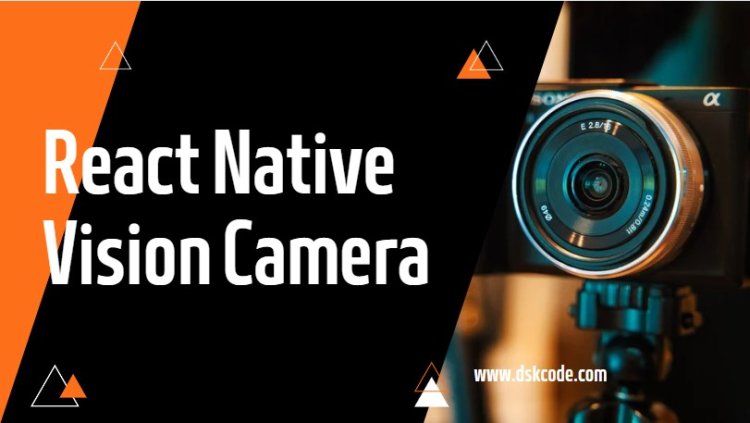
1. First, you need to create a new React Native project. You can do this by running the following command in your terminal:
2. Next, you need to install the React Native Vision Camera module. You can do this by running the following command in your terminal:
3. Once the installation is complete, you need to link the module to your project. You can do this by running the following command in your terminal:
4. Now that you have the module installed and linked to your project, you can start using it in your code. Here is an example of how you can use the module to display a camera preview:
import { StyleSheet, View } from 'react-native';
import { Camera } from 'react-native-vision-camera';
export default function App() {
const [isCameraReady, setIsCameraReady] = useState(false);
useEffect(() => {
(async () => {
await Camera.requestCameraPermission();
setIsCameraReady(true);
})();
}, []);
return (
<View style={styles.container}>
{isCameraReady ? <Camera style={styles.camera} / > : null}
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#000',
},
camera: {
flex: 1,
},
});
5. You can also use the module to capture photos or record videos. Here is an example of how you can capture a photo using the module:
import { StyleSheet, View, Button } from 'react-native';
import { Camera } from 'react-native-vision-camera';
import { useCameraDevices } from 'react-native-vision-camera';
export default function App() {
const [isCameraReady, setIsCameraReady] = useState(false);
const [camera, setCamera] = useState(null);
const [photoUri, setPhotoUri] = useState(null);
const { devices } = useCameraDevices();
useEffect(() => {
(async () => {
await Camera.requestCameraPermission();
setIsCameraReady(true);
})();
}, []);
const takePhoto = async () => {
const photo = await camera.takePhoto({ quality: 'max' });
setPhotoUri(photo.uri);
};
return (
<View style={styles.container}>
{isCameraReady ? (
<>
<Camera
style={styles.camera}
device={devices.back}
isActive={true}
onInitialized={(camera) => setCamera(camera)}
/>
<Button title="Take Photo" onPress={takePhoto} />
</>
) : null}
{photoUri ? (
<Image source={{ uri: photoUri }} style={styles.preview} />
) : null}
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#000',
},
camera: {
flex: 1,
},
preview: {
flex: 1,
width: '100%',
height: 300,
},
});
In this example, we use the `useCameraDevices` hook to get a list of available camera devices and the `takePhoto` method of the `Camera` component to capture a photo. We also use the `useState` hook to manage the state of the photo URI and display the captured photo in an `Image` component.
6. Finally, you can use the module to record a video. Here is an example of how you can record a video using the module:
import { StyleSheet, View, Button } from 'react-native';
import { Camera } from 'react-native-vision-camera';
import { useCameraDevices } from 'react-native-vision-camera';
export default function App() {
const [isCameraReady, setIsCameraReady] = useState(false);
const [camera, setCamera] = useState(null);
const [isRecording, setIsRecording] = useState(false);
const [videoUri, setVideoUri] = useState(null);
const { devices } = useCameraDevices();
useEffect(() => {
(async () => {
await Camera.requestCameraPermission();
setIsCameraReady(true);
})();
}, []);
const startRecording = async () => {
setIsRecording(true);
const video = await camera.record({ quality: 'max' });
setVideoUri(video.uri);
};
const stopRecording = () => {
setIsRecording(false);
camera.stopRecording();
};
return (
<View style={styles.container}>
{isCameraReady ? (
<>
<Camera
style={styles.camera}
device={devices.back}
isActive={true}
onInitialized={(camera) => setCamera(camera)}
/>
{isRecording ? (
<Button title="Stop Recording" onPress={stopRecording} />
) : (
<Button title="Start Recording" onPress={startRecording} />
)}
</>
) : null}
{videoUri ? (
<Video source={{ uri: videoUri }} style={styles.preview} />
) : null}
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#000',
},
camera: {
flex: 1,
},
preview: {
width: '100%',
height: 300,
},
});
In this example, we use the record and stopRecording methods of the Camera component to record a video. We also use the useState hook to manage the state of the recording and display the recorded video in a Video component.
That's it! With these examples, you should be able to start using the React Native Vision Camera module in your own projects.