Harness the Power of React Hooks
Learn how to use React Hooks to create powerful and dynamic React applications. Discover the tools and techniques you need to create more efficient and effective React components. Unlock the potential of React Hooks to help you build your React projects faster.
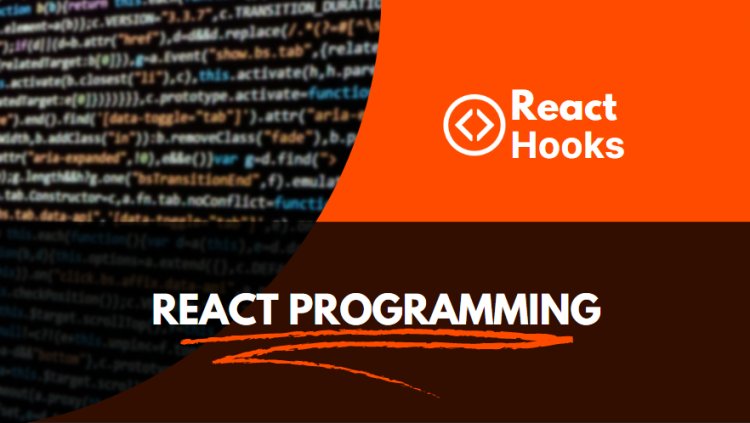
Table of Contents
1. Introduction to React Hooks
2. Benefits of Using React Hooks
3. How to Use React Hooks
4. Best Practices for React Hooks
5. React Hooks Limitations
6. React Hooks List
7. Troubleshooting React Hooks
Introduction to React Hooks
React Hooks are a new feature in React 16.8 that allow developers to use state and other React features without writing a class component. React Hooks enable developers to build more complex React applications that are easier to maintain, share, and debug. React Hooks also make it easier to share logic between components, allowing developers to keep their code DRY.
The main keywords associated with React Hooks are:
1. State: Hooks allow developers to use state in functional components, which was not possible before.
2. Functional Components: Hooks make it possible to use state and other features in functional components, making them more powerful than before.
3. Reusability: Hooks allow developers to share logic between components, making code more DRY and reusable.
4. Maintenance: Hooks make it easier to maintain React applications by allowing developers to share logic between components.
5. Debugging: Hooks make it easier to debug React applications, as the logic is shared between components.
Benefits of Using React Hooks
React Hooks provide several benefits over traditional React components, including improved performance, better code organization, and greater flexibility. By leveraging the power of React Hooks, developers can create components that are more reusable, more testable, and less complex.
React Hooks offer improved performance by allowing components to be written as functions. Traditional React components are written as classes, which can quickly become bloated due to the amount of boilerplate code required. With React Hooks, however, components can be written as functions, which are less verbose and can be optimized for better performance.
React Hooks also enable better code organization. With traditional React components, it can be difficult to keep track of all the different pieces of code needed to make a component work. With React Hooks, however, components can be organized better and the code can be more easily understood.
Finally, React Hooks provide greater flexibility. With traditional React components, developers are limited by the amount of code they can write. With React Hooks, however, developers can customize components with specific logic and behavior. This makes it easier to create custom components that can be used across multiple projects.
Overall, React Hooks offer developers a number of advantages over traditional React
How to Use React Hooks
React Hooks are a revolutionary new way to write React components. They make it possible to use state and other React features without writing a class. With React Hooks, you can manage state, perform side effects, and encapsulate reusable logic in your components.
To use React Hooks, you'll need to be familiar with the basic principles of React components. You'll also need to understand how to use JavaScript functions, as Hooks are just functions.
When you use React Hooks, you’re writing functions that return a React element. The React element is what you would normally write in a component's render method. When you use a Hook, the function is called with a single argument of an object containing the component's props.
To use a Hook, you'll need to import it from React. There are several built-in Hooks such as useState, useEffect, and useContext. You can also create custom Hooks to encapsulate component logic.
When you use a Hook, you need to pass it a set of arguments. The arguments can be anything you want, but they should be related to the component's props. For example, if you're creating a component to display a list of items
Best Practices for React Hooks
React Hooks are a powerful new way to work with React components. They offer a way to create components that are more reusable and more efficient than traditional React components. However, with this new way of working comes a set of best practices that should be followed to ensure a successful React Hooks implementation.
1. Only Call Hooks from React Functions: The first and most important rule of React Hooks is that Hooks must only be called from React functions. This means that Hooks should never be called from regular JavaScript functions, class components, or ES2015 classes. Hooks should only be called from React functional components.
2. Use Hooks at the Top Level: When calling Hooks, they should be called at the top level of the React function. Do not call Hooks inside loops, conditions, or nested functions. This will help ensure that Hooks are called in the correct order and prevent any unexpected behavior.
3. Only Call Hooks from React Functions: The first and most important rule of React Hooks is that Hooks must only be called from React functions. This means that Hooks should never be called from regular JavaScript functions, class components, or ES2015 classes. Hooks should only be called from
React Hooks Limitations
React Hooks are a new feature in React that provides a way to handle state and side effects in function components. While React Hooks provide a lot of advantages, they also have several limitations.
First, React Hooks must be called at the top level of a function component in order to work. This means that they cannot be used within a loop or nested inside of a condition. This can be a limitation when it comes to complex logic.
Second, React Hooks can only be used in function components and cannot be used in class components. This can be a problem if you need to use a class component for a particular feature or if you want to reuse a existing class component.
Third, React Hooks cannot be used to access lifecycle methods, like componentDidMount or componentDidUpdate. This can be a limitation if you need to perform a certain task when a component mounts or updates.
Fourth, React Hooks can only be used with React components, so they cannot be used outside of a React app. This can be a limitation if you need to use a third-party library or write custom logic in a non-React environment.
React Hooks List
React provides several built-in hooks that you can use in your components:
• useState
• useEffect
• useContext
• useReducer
• useMemo
• useRef
• useCallback
• useImperativeHandle
• useLayoutEffect
• useDebugValue
Troubleshooting React Hooks
React Hooks are a powerful new feature introduced in React 16.8 that allow you to use state and other React features without writing a class. They enable you to use functional components instead of classes when creating React components. While React Hooks can be a great addition to your components, they can also be tricky to troubleshoot. In this article, we will discuss some tips for troubleshooting React Hooks.
The first step in troubleshooting React Hooks is to understand what they are and how they work. React Hooks are functions that allow you to access React features such as state and lifecycle methods from within a functional component. They are designed to make it easier to write components that can interact with the React features without having to use classes.
When troubleshooting React Hooks, it is important to remember that they are still relatively new and may not always work as expected. If you are having trouble getting a particular hook to work, it is important to check the documentation to make sure you are using it correctly.